Connectomics
[ ]:
# Install all used libraries
!pip install -r requirements.txt --upgrade
[ ]:
# Import libs and initialise API objects
from vfb_connect.cross_server_tools import VfbConnect
import pandas as pd
vc = VfbConnect()
import pymaid
import navis
navis.set_pbars(jupyter=False)
pymaid.set_pbars(jupyter=False)
# Connect to VFB's CATMAID server hosting the FAFB data
rm = pymaid.connect_catmaid(server="https://fafb.catmaid.virtualflybrain.org/", api_token=None, max_threads=10)
# Test call to see if connection works
print(f'Server is running CATMAID version {rm.catmaid_version}')
WARNING: Could not load OpenGL library.
INFO : Global CATMAID instance set. Caching is ON. (pymaid)
Server is running CATMAID version 2020.02.15-990-gb5aac7433
VFB provides a simple methods for exploring connectomics data
get_connected_neurons_by_type
allows queries for connections between defined upstream and downstream neuron types/classes. This supports a level of abstraction not available without VFB, where grouping of neurons by higher level classifications is limited to extraction of classification from standardised names. The following examples take advantage of the abstract clasifications on VFB to explore direct synaptic connections.
[ ]:
neurons_in_DA3 = vc.get_instances("'neuron' that 'overlaps' some 'antennal lobe glomerulus DA3'", summary=True)
neurons_in_DA3
Running query: FBbt:00005106 that RO:0002131 some FBbt:00003934
Query URL: http://owl.virtualflybrain.org/kbs/vfb/instances?object=FBbt%3A00005106+that+RO%3A0002131+some+FBbt%3A00003934&prefixes=%7B%22FBbt%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FFBbt_%22%2C+%22RO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FRO_%22%2C+%22BFO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FBFO_%22%7D&direct=False
Query results: 158
[{'label': 'ORN_DL3_R (FlyEM-HB:1671625186)',
'symbol': '',
'id': 'VFB_jrchk1hj',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or65',
'parents_id': 'FBbt_00067011',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1671625186',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DL4_L (FlyEM-HB:1640257586)',
'symbol': '',
'id': 'VFB_jrchk1ki',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or49a/85f',
'parents_id': 'FBbt_00067055',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1640257586',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'DL4_adPN_R (FlyEM-HB:1670934213)',
'symbol': '',
'id': 'VFB_jrchjteh',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Cholinergic|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe projection neuron DL4 adPN',
'parents_id': 'FBbt_00100382',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1670934213',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1796080038)',
'symbol': '',
'id': 'VFB_jrchk15w',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1796080038',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1735059028)',
'symbol': '',
'id': 'VFB_jrchk18f',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735059028',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1735408330)',
'symbol': '',
'id': 'VFB_jrchk18s',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735408330',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DL3_L (FlyEM-HB:1640572862)',
'symbol': '',
'id': 'VFB_jrchk1il',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or65',
'parents_id': 'FBbt_00067011',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1640572862',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_VA6 (FlyEM-HB:1921989317)',
'symbol': '',
'id': 'VFB_jrchk2ao',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or82a',
'parents_id': 'FBbt_00067044',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1921989317',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2F_b(Full)_R (FlyEM-HB:1640909284)',
'symbol': '',
'id': 'VFB_jrchk8am',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2F',
'parents_id': 'FBbt_00049812',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1640909284',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA4m (FlyEM-HB:1796792278)',
'symbol': '',
'id': 'VFB_jrchk19g',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or2a',
'parents_id': 'FBbt_00067001',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1796792278',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1672311674)',
'symbol': '',
'id': 'VFB_jrchk17v',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1672311674',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:1735391275)',
'symbol': '',
'id': 'VFB_jrchk14f',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735391275',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734717736)',
'symbol': '',
'id': 'VFB_jrchk17w',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734717736',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1735399719)',
'symbol': '',
'id': 'VFB_jrchk18g',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735399719',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1734691780)',
'symbol': '',
'id': 'VFB_jrchk134',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734691780',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1735059089)',
'symbol': '',
'id': 'VFB_jrchk18r',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735059089',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'v2LN3b_R (FlyEM-HB:1888199872)',
'symbol': '',
'id': 'VFB_jrchk8fg',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 3B v2LN',
'parents_id': 'FBbt_20003755',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1888199872',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1735059070)',
'symbol': '',
'id': 'VFB_jrchk18q',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735059070',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1735050861)',
'symbol': '',
'id': 'VFB_jrchk15b',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735050861',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_D (FlyEM-HB:1605204164)',
'symbol': '',
'id': 'VFB_jrchk128',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or69a',
'parents_id': 'FBbt_00067034',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1605204164',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2S(Star)_R (FlyEM-HB:1702306037)',
'symbol': '',
'id': 'VFB_jrchk8b7',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2S',
'parents_id': 'FBbt_00049815',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1702306037',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1703342190)',
'symbol': '',
'id': 'VFB_jrchk12z',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703342190',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734182300)',
'symbol': '',
'id': 'VFB_jrchk17x',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734182300',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN11_R (FlyEM-HB:2040301572)',
'symbol': '',
'id': 'VFB_jrchk89d',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 11 lLN',
'parents_id': 'FBbt_20003745',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '2040301572',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_D (FlyEM-HB:5812993853)',
'symbol': '',
'id': 'VFB_jrchk12n',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or69a',
'parents_id': 'FBbt_00067034',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5812993853',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734717804)',
'symbol': '',
'id': 'VFB_jrchk187',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734717804',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DL3_R (FlyEM-HB:1672302763)',
'symbol': '',
'id': 'VFB_jrchk1hv',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or65',
'parents_id': 'FBbt_00067011',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1672302763',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DL5_L (FlyEM-HB:1640050851)',
'symbol': '',
'id': 'VFB_jrchk1lh',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or7a',
'parents_id': 'FBbt_00067005',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1640050851',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'Uniglomerular mALT DA3 adPN#L2 (FAFB:2350853)',
'symbol': '',
'id': 'VFB_0010122u',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Cholinergic|Individual|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe projection neuron DA3 adPN',
'parents_id': 'FBbt_00100384',
'data_source': 'catmaid_fafb',
'accession': '2350853',
'templates': 'JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2F_a(Full)_R (FlyEM-HB:5901218894)',
'symbol': '',
'id': 'VFB_jrchk8ak',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2F',
'parents_id': 'FBbt_00049812',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5901218894',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1703683340)',
'symbol': '',
'id': 'VFB_jrchk186',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703683340',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DC1_L (FlyEM-HB:1605198915)',
'symbol': '',
'id': 'VFB_jrchk19z',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or19',
'parents_id': 'FBbt_00067018',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1605198915',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_c_R (FlyEM-HB:5813047691)',
'symbol': '',
'id': 'VFB_jrchk8ae',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813047691',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'Uniglomerular mALT DA3 adPN#L1 (FAFB:2449792)',
'symbol': '',
'id': 'VFB_0010123h',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Cholinergic|Individual|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe projection neuron DA3 adPN',
'parents_id': 'FBbt_00100384',
'data_source': 'catmaid_fafb',
'accession': '2449792',
'templates': 'JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'M_vPNml50_R (FlyEM-HB:610274029)',
'symbol': '',
'id': 'VFB_jrchk0xz',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult multiglomerular antennal lobe projection neuron type 50 vPN',
'parents_id': 'FBbt_20003827',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '610274029',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1703151910)',
'symbol': '',
'id': 'VFB_jrchk185',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703151910',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_a(Tortuous)_R (FlyEM-HB:5813055277)',
'symbol': '',
'id': 'VFB_jrchk8bf',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813055277',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DC3 (FlyEM-HB:1797815029)',
'symbol': '',
'id': 'VFB_jrchk1bf',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or83c',
'parents_id': 'FBbt_00067047',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1797815029',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734713762)',
'symbol': '',
'id': 'VFB_jrchk18a',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734713762',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'D_adPN_R (FlyEM-HB:5813055184)',
'symbol': '',
'id': 'VFB_jrchjti8',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Cholinergic|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe projection neuron D adPN',
'parents_id': 'FBbt_00067355',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813055184',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:1703683156)',
'symbol': '',
'id': 'VFB_jrchk13f',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703683156',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1766409090)',
'symbol': '',
'id': 'VFB_jrchk13a',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1766409090',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_b_R (FlyEM-HB:5813069055)',
'symbol': '',
'id': 'VFB_jrchk8aa',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813069055',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_a(Tortuous)_R (FlyEM-HB:1762354941)',
'symbol': '',
'id': 'VFB_jrchk8bg',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1762354941',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_b_R (FlyEM-HB:5813054777)',
'symbol': '',
'id': 'VFB_jrchk8a9',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813054777',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_b_R (FlyEM-HB:5813054622)',
'symbol': '',
'id': 'VFB_jrchk8a8',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813054622',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:1703682799)',
'symbol': '',
'id': 'VFB_jrchk15e',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703682799',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1735404269)',
'symbol': '',
'id': 'VFB_jrchk18t',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735404269',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'l2LN18_R (FlyEM-HB:5813054773)',
'symbol': '',
'id': 'VFB_jrchk891',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 18 l2LN',
'parents_id': 'FBbt_20003734',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813054773',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:1797439276)',
'symbol': '',
'id': 'VFB_jrchk160',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1797439276',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_b_R (FlyEM-HB:1642623277)',
'symbol': '',
'id': 'VFB_jrchk8ad',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1642623277',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_e(Tortuous)_R (FlyEM-HB:1640922516)',
'symbol': '',
'id': 'VFB_jrchk8bs',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1640922516',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2S(Star)_R (FlyEM-HB:1640922754)',
'symbol': '',
'id': 'VFB_jrchk8b8',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2S',
'parents_id': 'FBbt_00049815',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1640922754',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2P_a(Patchy)_R (FlyEM-HB:2041621893)',
'symbol': '',
'id': 'VFB_jrchk8ao',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2P',
'parents_id': 'FBbt_00049813',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '2041621893',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'Uniglomerular mALT DA3 adPN#R1 (FAFB:57349)',
'symbol': '',
'id': 'VFB_00101239',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Cholinergic|Individual|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe projection neuron DA3 adPN',
'parents_id': 'FBbt_00100384',
'data_source': 'catmaid_fafb',
'accession': '57349',
'templates': 'JRC2018Unisex|adult brain template JFRC2',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1734350882)',
'symbol': '',
'id': 'VFB_jrchk15c',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734350882',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DM3_L (FlyEM-HB:1543124896)',
'symbol': '',
'id': 'VFB_jrchk1qn',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or47a',
'parents_id': 'FBbt_00067054',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1543124896',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:1735032816)',
'symbol': '',
'id': 'VFB_jrchk14l',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735032816',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1733850183)',
'symbol': '',
'id': 'VFB_jrchk182',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1733850183',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2F_b(Full)_R (FlyEM-HB:5813024698)',
'symbol': '',
'id': 'VFB_jrchk8an',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2F',
'parents_id': 'FBbt_00049812',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813024698',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734718175)',
'symbol': '',
'id': 'VFB_jrchk18h',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734718175',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3_R (FlyEM-HB:1703683060)',
'symbol': '',
'id': 'VFB_jrchk183',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703683060',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'M_lvPNm47_R (FlyEM-HB:1856820042)',
'symbol': '',
'id': 'VFB_jrchk0xq',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult multiglomerular antennal lobe projection neuron type 47 lvPN',
'parents_id': 'FBbt_20003826',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1856820042',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'M_vPNml50_R (FlyEM-HB:610274046)',
'symbol': '',
'id': 'VFB_jrchk0y0',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult multiglomerular antennal lobe projection neuron type 50 vPN',
'parents_id': 'FBbt_20003827',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '610274046',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'OA-VUMa5_R (FlyEM-HB:2069652905)',
'symbol': '',
'id': 'VFB_jrchk10l',
'tags': 'Entity|Octopaminergic|Adult|Anatomy|has_image|Cell|Individual|has_neuron_connectivity|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'octopaminergic VUMa5 neuron',
'parents_id': 'FBbt_00047934',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '2069652905',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_b(Tortuous)_R (FlyEM-HB:5813034493)',
'symbol': '',
'id': 'VFB_jrchk8bm',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813034493',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734717977)',
'symbol': '',
'id': 'VFB_jrchk18p',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734717977',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1735054729)',
'symbol': '',
'id': 'VFB_jrchk18c',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735054729',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:1734355428)',
'symbol': '',
'id': 'VFB_jrchk14w',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734355428',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_D (FlyEM-HB:1639368236)',
'symbol': '',
'id': 'VFB_jrchk121',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or69a',
'parents_id': 'FBbt_00067034',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1639368236',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_VA1d (FlyEM-HB:1921933455)',
'symbol': '',
'id': 'VFB_jrchk1zh',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or88a',
'parents_id': 'FBbt_00067057',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1921933455',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'v2LN3b_R (FlyEM-HB:5813034455)',
'symbol': '',
'id': 'VFB_jrchk8ff',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 3B v2LN',
'parents_id': 'FBbt_20003755',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813034455',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:1672315788)',
'symbol': '',
'id': 'VFB_jrchk13i',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1672315788',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_b(Tortuous)_R (FlyEM-HB:1640572741)',
'symbol': '',
'id': 'VFB_jrchk8bl',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1640572741',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_c(Tortuous)_R (FlyEM-HB:1671292719)',
'symbol': '',
'id': 'VFB_jrchk8bo',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1671292719',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2P_b(Patchy)_R (FlyEM-HB:1946178096)',
'symbol': '',
'id': 'VFB_jrchk8au',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2P',
'parents_id': 'FBbt_00049813',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1946178096',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1671970212)',
'symbol': '',
'id': 'VFB_jrchk163',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1671970212',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2R_b(Regional)_R (FlyEM-HB:1702305987)',
'symbol': '',
'id': 'VFB_jrchk8b5',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2R',
'parents_id': 'FBbt_00049814',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1702305987',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734718209)',
'symbol': '',
'id': 'VFB_jrchk18j',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734718209',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DL4_R (FlyEM-HB:1671271059)',
'symbol': '',
'id': 'VFB_jrchk1km',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or49a/85f',
'parents_id': 'FBbt_00067055',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1671271059',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_c_R (FlyEM-HB:1702651358)',
'symbol': '',
'id': 'VFB_jrchk8aj',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1702651358',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1735058916)',
'symbol': '',
'id': 'VFB_jrchk18d',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735058916',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_b_R (FlyEM-HB:1610530558)',
'symbol': '',
'id': 'VFB_jrchk8ac',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1610530558',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3_R (FlyEM-HB:1701960169)',
'symbol': '',
'id': 'VFB_jrchk17z',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1701960169',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DL3_L (FlyEM-HB:1606205114)',
'symbol': '',
'id': 'VFB_jrchk1hb',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or65',
'parents_id': 'FBbt_00067011',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1606205114',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_c_R (FlyEM-HB:1547454812)',
'symbol': '',
'id': 'VFB_jrchk8ai',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1547454812',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1703342127)',
'symbol': '',
'id': 'VFB_jrchk180',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703342127',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1574160129)',
'symbol': '',
'id': 'VFB_jrchk13s',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1574160129',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734377152)',
'symbol': '',
'id': 'VFB_jrchk18i',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734377152',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1703678670)',
'symbol': '',
'id': 'VFB_jrchk15v',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703678670',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_VA1v (FlyEM-HB:2075398249)',
'symbol': '',
'id': 'VFB_jrchk22d',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or47b',
'parents_id': 'FBbt_00067056',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '2075398249',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN11_R (FlyEM-HB:1670278227)',
'symbol': '',
'id': 'VFB_jrchk89e',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 11 lLN',
'parents_id': 'FBbt_20003745',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1670278227',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_c_R (FlyEM-HB:1824101645)',
'symbol': '',
'id': 'VFB_jrchk8ag',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1824101645',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_d(Tortuous)_R (FlyEM-HB:1667251683)',
'symbol': '',
'id': 'VFB_jrchk8bp',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1667251683',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:5813020396)',
'symbol': '',
'id': 'VFB_jrchk13k',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813020396',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DL5_L (FlyEM-HB:1669933011)',
'symbol': '',
'id': 'VFB_jrchk1li',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or7a',
'parents_id': 'FBbt_00067005',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1669933011',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1735063134)',
'symbol': '',
'id': 'VFB_jrchk18e',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735063134',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_a_R (FlyEM-HB:5813130064)',
'symbol': '',
'id': 'VFB_jrchk8a4',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813130064',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3_R (FlyEM-HB:1734713691)',
'symbol': '',
'id': 'VFB_jrchk18n',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734713691',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_a(Tortuous)_R (FlyEM-HB:5813054726)',
'symbol': '',
'id': 'VFB_jrchk8bh',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813054726',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN12a_R (FlyEM-HB:1826445251)',
'symbol': '',
'id': 'VFB_jrchk89g',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 12A lLN',
'parents_id': 'FBbt_20003746',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1826445251',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_b_R (FlyEM-HB:5813078440)',
'symbol': '',
'id': 'VFB_jrchk8a7',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813078440',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1702811119)',
'symbol': '',
'id': 'VFB_jrchk181',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1702811119',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734377183)',
'symbol': '',
'id': 'VFB_jrchk18k',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734377183',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:1735382530)',
'symbol': '',
'id': 'VFB_jrchk14h',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735382530',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'DA3_adPN_R (FlyEM-HB:666135689)',
'symbol': '',
'id': 'VFB_jrchjtdo',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Cholinergic|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe projection neuron DA3 adPN',
'parents_id': 'FBbt_00100384',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '666135689',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'v2LN35_R (FlyEM-HB:1733056086)',
'symbol': '',
'id': 'VFB_jrchk8ew',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 35 v2LN',
'parents_id': 'FBbt_20003766',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1733056086',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734713656)',
'symbol': '',
'id': 'VFB_jrchk188',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734713656',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_b_R (FlyEM-HB:1640887603)',
'symbol': '',
'id': 'VFB_jrchk8a6',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1640887603',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_b_R (FlyEM-HB:5813054725)',
'symbol': '',
'id': 'VFB_jrchk8ab',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813054725',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1672311578)',
'symbol': '',
'id': 'VFB_jrchk13h',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1672311578',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'M_spPN5t10_R (FlyEM-HB:1733370882)',
'symbol': '',
'id': 'VFB_jrchk0xy',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult multiglomerular antennal lobe projection neuron spPN t5ALT',
'parents_id': 'FBbt_00051066',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1733370882',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'M_vPNml53_R (FlyEM-HB:5813008771)',
'symbol': '',
'id': 'VFB_jrchk0y3',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult multiglomerular antennal lobe projection neuron type 53 vPN',
'parents_id': 'FBbt_20003830',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813008771',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2F_a(Full)_R (FlyEM-HB:1670287030)',
'symbol': '',
'id': 'VFB_jrchk8al',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2F',
'parents_id': 'FBbt_00049812',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1670287030',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_e(Tortuous)_R (FlyEM-HB:1699974843)',
'symbol': '',
'id': 'VFB_jrchk8br',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1699974843',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2S(Star)_R (FlyEM-HB:1762359683)',
'symbol': '',
'id': 'VFB_jrchk8bc',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2S',
'parents_id': 'FBbt_00049815',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1762359683',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'v2LN30_R (FlyEM-HB:1671620613)',
'symbol': '',
'id': 'VFB_jrchk8e8',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 30 v2LN',
'parents_id': 'FBbt_20003756',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1671620613',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DM3_L (FlyEM-HB:1636225517)',
'symbol': '',
'id': 'VFB_jrchk1qy',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or47a',
'parents_id': 'FBbt_00067054',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1636225517',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1704028333)',
'symbol': '',
'id': 'VFB_jrchk18l',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1704028333',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2S(Star)_R (FlyEM-HB:5813069085)',
'symbol': '',
'id': 'VFB_jrchk8b9',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2S',
'parents_id': 'FBbt_00049815',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813069085',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN16b_R (FlyEM-HB:1887168462)',
'symbol': '',
'id': 'VFB_jrchk89w',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 16B lLN',
'parents_id': 'FBbt_20003752',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1887168462',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_a(Tortuous)_R (FlyEM-HB:5813032595)',
'symbol': '',
'id': 'VFB_jrchk8bd',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813032595',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1672643988)',
'symbol': '',
'id': 'VFB_jrchk143',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1672643988',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2T_a(Tortuous)_R (FlyEM-HB:5813056598)',
'symbol': '',
'id': 'VFB_jrchk8bi',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2T',
'parents_id': 'FBbt_00049816',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813056598',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:1735395524)',
'symbol': '',
'id': 'VFB_jrchk13p',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735395524',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1670972802)',
'symbol': '',
'id': 'VFB_jrchk184',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1670972802',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'OA-VUMa2_R (FlyEM-HB:1670265016)',
'symbol': '',
'id': 'VFB_jrchk10i',
'tags': 'Entity|Octopaminergic|Adult|Anatomy|has_image|Cell|Individual|has_neuron_connectivity|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'octopaminergic VUMa2 neuron',
'parents_id': 'FBbt_00110157',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1670265016',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2S(Star)_R (FlyEM-HB:1670627928)',
'symbol': '',
'id': 'VFB_jrchk8bb',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2S',
'parents_id': 'FBbt_00049815',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1670627928',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN10_R (FlyEM-HB:1825789179)',
'symbol': '',
'id': 'VFB_jrchk89b',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 10 lLN',
'parents_id': 'FBbt_20003744',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1825789179',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734718131)',
'symbol': '',
'id': 'VFB_jrchk18m',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734718131',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1734722060)',
'symbol': '',
'id': 'VFB_jrchk18b',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1734722060',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1735399997)',
'symbol': '',
'id': 'VFB_jrchk18o',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735399997',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'DA4m_adPN_R (FlyEM-HB:574037266)',
'symbol': '',
'id': 'VFB_jrchjtdq',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Cholinergic|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe projection neuron DA4m adPN',
'parents_id': 'FBbt_00047714',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '574037266',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1766434854)',
'symbol': '',
'id': 'VFB_jrchk18u',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1766434854',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'M_l2PNl21_R (FlyEM-HB:1700307582)',
'symbol': '',
'id': 'VFB_jrchk0w1',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult multiglomerular antennal lobe projection neuron type 21 l2PN',
'parents_id': 'FBbt_20003797',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1700307582',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1703147500)',
'symbol': '',
'id': 'VFB_jrchk17y',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703147500',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_R (FlyEM-HB:1735054607)',
'symbol': '',
'id': 'VFB_jrchk13v',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1735054607',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2R_b(Regional)_R (FlyEM-HB:5813076969)',
'symbol': '',
'id': 'VFB_jrchk8b6',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2R',
'parents_id': 'FBbt_00049814',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813076969',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN2S(Star)_R (FlyEM-HB:1732995501)',
'symbol': '',
'id': 'VFB_jrchk8ba',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2S',
'parents_id': 'FBbt_00049815',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1732995501',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_c_R (FlyEM-HB:1578826464)',
'symbol': '',
'id': 'VFB_jrchk8ah',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1578826464',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA4l (FlyEM-HB:1828168256)',
'symbol': '',
'id': 'VFB_jrchk18v',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or43a',
'parents_id': 'FBbt_00067048',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1828168256',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN16a_R (FlyEM-HB:1702318692)',
'symbol': '',
'id': 'VFB_jrchk89t',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 16A lLN',
'parents_id': 'FBbt_20003751',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1702318692',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA2 (FlyEM-HB:1702107389)',
'symbol': '',
'id': 'VFB_jrchk16z',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or56a',
'parents_id': 'FBbt_00067063',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1702107389',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DL5_R (FlyEM-HB:1639925326)',
'symbol': '',
'id': 'VFB_jrchk1lb',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or7a',
'parents_id': 'FBbt_00067005',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1639925326',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'CSD(5HT1)(AVM03)_L (FlyEM-HB:759810119)',
'symbol': '',
'id': 'VFB_jrchjtd6',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Serotonergic|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult CSD interneuron',
'parents_id': 'FBbt_00007405',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '759810119',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN2P_c(Patchy)_R (FlyEM-HB:2105086391)',
'symbol': '',
'id': 'VFB_jrchk8b0',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe lateral local neuron 2P',
'parents_id': 'FBbt_00049813',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '2105086391',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'il3LN6_R (FlyEM-HB:5813018460)',
'symbol': '',
'id': 'VFB_jrchk88v',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'antennal lobe local neuron 6',
'parents_id': 'FBbt_00047710',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813018460',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1764703552)',
'symbol': '',
'id': 'VFB_jrchk146',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1764703552',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'DA3_adPN_R (FlyEM-HB:1703683361)',
'symbol': '',
'id': 'VFB_jrchjtdn',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Cholinergic|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe projection neuron DA3 adPN',
'parents_id': 'FBbt_00100384',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703683361',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DM3_L (FlyEM-HB:1667592552)',
'symbol': '',
'id': 'VFB_jrchk1qs',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or47a',
'parents_id': 'FBbt_00067054',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1667592552',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DL3_L (FlyEM-HB:1640922302)',
'symbol': '',
'id': 'VFB_jrchk1gx',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or65',
'parents_id': 'FBbt_00067011',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1640922302',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'lLN1_c_R (FlyEM-HB:5813062199)',
'symbol': '',
'id': 'VFB_jrchk8af',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe local neuron type 1 lLN',
'parents_id': 'FBbt_20003740',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '5813062199',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'Uniglomerular mALT DA3 adPN#R2 (FAFB:65465)',
'symbol': '',
'id': 'VFB_00101240',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Cholinergic|Individual|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult antennal lobe projection neuron DA3 adPN',
'parents_id': 'FBbt_00100384',
'data_source': 'catmaid_fafb',
'accession': '65465',
'templates': 'JRC2018Unisex|adult brain template JFRC2',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DL4_L (FlyEM-HB:1670623474)',
'symbol': '',
'id': 'VFB_jrchk1jn',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or49a/85f',
'parents_id': 'FBbt_00067055',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1670623474',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA1_L (FlyEM-HB:1764703462)',
'symbol': '',
'id': 'VFB_jrchk15p',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or67d',
'parents_id': 'FBbt_00067031',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1764703462',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA4m (FlyEM-HB:1827840165)',
'symbol': '',
'id': 'VFB_jrchk19f',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|NBLAST|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or2a',
'parents_id': 'FBbt_00067001',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1827840165',
'templates': 'JRC_FlyEM_Hemibrain|JRC2018Unisex',
'dataset': 'None',
'license': ''},
{'label': 'ORN_DA3 (FlyEM-HB:1703683329)',
'symbol': '',
'id': 'VFB_jrchk189',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or23a',
'parents_id': 'FBbt_00067033',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1703683329',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''},
{'label': 'ORN_VA1d (FlyEM-HB:1951616602)',
'symbol': '',
'id': 'VFB_jrchk1zl',
'tags': 'Entity|has_image|Adult|Anatomy|has_neuron_connectivity|Cell|Individual|Sensory_neuron|has_region_connectivity|Nervous_system|Neuron',
'parents_label': 'adult olfactory receptor neuron Or88a',
'parents_id': 'FBbt_00067057',
'data_source': 'neuprint_JRC_Hemibrain_1point1',
'accession': '1951616602',
'templates': 'JRC2018Unisex|JRC_FlyEM_Hemibrain',
'dataset': 'None',
'license': ''}]
[ ]:
# Find all GABA-regic inputs onto descending neurons
vc.get_connected_neurons_by_type(upstream_type='GABAergic neuron',
downstream_type='adult descending neuron',
weight=10).sort_values('weight', ascending=False, ignore_index=True)
upstream_neuron_id | upstream_neuron_name | weight | downstream_neuron_id | downstream_neuron_name | upstream_class | downstream_class | up_data_source | up_accession | down_source | down_accession | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | VFB_001011rg | Uniglomerular mlALT VP4 vPN bilateral#R1 (FAFB... | 156 | VFB_001011rk | DNp44 (FAFB:3094186) | adult antennal lobe projection neuron VP4+ vPN | descending neuron of the posterior brain DNp44... | None | None | None | None |
1 | VFB_00101152 | Uniglomerular t5-mlALT VP4 vPN#R1 (FAFB:1149173) | 142 | VFB_001011rk | DNp44 (FAFB:3094186) | adult antennal lobe projection neuron VP4 vPN | descending neuron of the posterior brain DNp44... | None | None | None | None |
2 | VFB_jrchk7ji | VP4_vPN(mlALT)_R (FlyEM-HB:634759240) | 65 | VFB_jrchjthu | DNp44_R (FlyEM-HB:542751938) | adult antennal lobe projection neuron VP4 vPN | descending neuron of the posterior brain DNp44 | None | None | None | None |
3 | VFB_jrchk7jh | VP4+_vPN(mlALT)_R (FlyEM-HB:543010474) | 50 | VFB_jrchjthu | DNp44_R (FlyEM-HB:542751938) | adult antennal lobe projection neuron VP4+ vPN | descending neuron of the posterior brain DNp44 | None | None | None | None |
4 | VFB_jrchk7jh | VP4+_vPN(mlALT)_R (FlyEM-HB:543010474) | 47 | VFB_jrchjthj | DNp25_R (FlyEM-HB:451689001) | adult antennal lobe projection neuron VP4+ vPN | descending neuron of the posterior brain DNp25 | None | None | None | None |
5 | VFB_jrchk8dp | oviIN_R (FlyEM-HB:423101189) | 43 | VFB_jrchk8dm | oviDNa_R (FlyEM-HB:550655668) | oviposition inhibitory neuron | oviposition descending neuron a | None | None | None | None |
6 | VFB_jrchk8dq | oviIN_L (FlyEM-HB:485934965) | 40 | VFB_jrchk8do | oviDNb_R (FlyEM-HB:519949044) | oviposition inhibitory neuron | oviposition descending neuron b | None | None | None | None |
7 | VFB_jrchk8dp | oviIN_R (FlyEM-HB:423101189) | 36 | VFB_jrchk8do | oviDNb_R (FlyEM-HB:519949044) | oviposition inhibitory neuron | oviposition descending neuron b | None | None | None | None |
8 | VFB_jrchk8dp | oviIN_R (FlyEM-HB:423101189) | 35 | VFB_jrchk8dn | oviDNb(PDM15)_L (FlyEM-HB:642763374) | oviposition inhibitory neuron | oviposition descending neuron b | None | None | None | None |
9 | VFB_001013vv | oviIN (FAFB:13325634) | 35 | VFB_001013vm | oviDNa#2 (FAFB:1875104) | oviposition inhibitory neuron | oviposition descending neuron a | None | None | None | None |
10 | VFB_jrchk8dq | oviIN_L (FlyEM-HB:485934965) | 32 | VFB_jrchk8dn | oviDNb(PDM15)_L (FlyEM-HB:642763374) | oviposition inhibitory neuron | oviposition descending neuron b | None | None | None | None |
11 | VFB_001013vv | oviIN (FAFB:13325634) | 29 | VFB_001013vl | oviDNb (FAFB:1862762) | oviposition inhibitory neuron | oviposition descending neuron b | None | None | None | None |
12 | VFB_jrchk7jh | VP4+_vPN(mlALT)_R (FlyEM-HB:543010474) | 28 | VFB_jrchjtg4 | DNg30_R (FlyEM-HB:571346836) | adult antennal lobe projection neuron VP4+ vPN | descending neuron of the gnathal ganglion DNg30 | None | None | None | None |
13 | VFB_jrchk8dq | oviIN_L (FlyEM-HB:485934965) | 27 | VFB_jrchk8dm | oviDNa_R (FlyEM-HB:550655668) | oviposition inhibitory neuron | oviposition descending neuron a | None | None | None | None |
14 | VFB_001013vv | oviIN (FAFB:13325634) | 20 | VFB_001013vt | oviDNa#1 (FAFB:5143346) | oviposition inhibitory neuron | oviposition descending neuron a | None | None | None | None |
15 | VFB_jrchjza4 | LHPV2c1_b_R (FlyEM-HB:825032139) | 13 | VFB_jrchjthr | DNp32_R (FlyEM-HB:5813050455) | adult lateral horn PV2c1 neuron | descending neuron of the posterior brain DNp32 | None | None | None | None |
16 | VFB_jrchjtd8 | CT1_L (FlyEM-HB:1311993208) | 12 | VFB_jrchjtgf | DNp11_R (FlyEM-HB:1281324958) | tangential neuron CT1 | descending neuron of the posterior brain DNp11 | None | None | None | None |
17 | VFB_jrchk7jh | VP4+_vPN(mlALT)_R (FlyEM-HB:543010474) | 12 | VFB_jrchjthh | DNp24_R (FlyEM-HB:5813047199) | adult antennal lobe projection neuron VP4+ vPN | descending neuron of the posterior brain DNp24 | None | None | None | None |
18 | VFB_jrchjza6 | LHPV2c1_c_R (FlyEM-HB:913345831) | 12 | VFB_jrchjthr | DNp32_R (FlyEM-HB:5813050455) | adult lateral horn PV2c1 neuron | descending neuron of the posterior brain DNp32 | None | None | None | None |
19 | VFB_jrchjza3 | LHPV2c1_b_R (FlyEM-HB:856066807) | 11 | VFB_jrchjthr | DNp32_R (FlyEM-HB:5813050455) | adult lateral horn PV2c1 neuron | descending neuron of the posterior brain DNp32 | None | None | None | None |
20 | VFB_jrchjymc | LHAV1a3_R (FlyEM-HB:823999645) | 10 | VFB_jrchjtht | DNp42_R (FlyEM-HB:981000564) | adult lateral horn AV1a3 neuron | descending neuron of the posterior brain | None | None | None | None |
21 | VFB_jrchk7ji | VP4_vPN(mlALT)_R (FlyEM-HB:634759240) | 10 | VFB_jrchjtg4 | DNg30_R (FlyEM-HB:571346836) | adult antennal lobe projection neuron VP4 vPN | descending neuron of the gnathal ganglion DNg30 | None | None | None | None |
22 | VFB_jrchjywh | LHAV4c2_R (FlyEM-HB:921969630) | 10 | VFB_jrchk8rz | vpoDN_R (FlyEM-HB:5813057864) | adult lateral horn AV4c2 neuron | adult doublesex pMN2 (female) neuron | None | None | None | None |
[ ]:
# Get connections between pacemaker neurons
clock_neuron_connectome = vc.get_connected_neurons_by_type(upstream_type='pacemaker neuron',
downstream_type='pacemaker neuron',
weight=10).sort_values('weight', ascending=False)
clock_neuron_connectome
upstream_neuron_id | upstream_neuron_name | weight | downstream_neuron_id | downstream_neuron_name | upstream_class | downstream_class | up_data_source | up_accession | down_source | down_accession | |
---|---|---|---|---|---|---|---|---|---|---|---|
14 | VFB_jrchjtex | DN1a_R (FlyEM-HB:264083994) | 79 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | DN1a neuron | LNd neuron | None | None | None | None |
0 | VFB_jrchjtey | DN1a_R (FlyEM-HB:5813022274) | 75 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | DN1a neuron | LNd neuron | None | None | None | None |
26 | VFB_jrchjtey | DN1a_R (FlyEM-HB:5813022274) | 63 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | DN1a neuron | s-LNv neuron | None | None | None | None |
31 | VFB_jrchjtex | DN1a_R (FlyEM-HB:264083994) | 55 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | DN1a neuron | s-LNv neuron | None | None | None | None |
7 | VFB_jrchk089 | LPN_R (FlyEM-HB:480029788) | 43 | VFB_jrchk08a | LPN_R (FlyEM-HB:450034902) | LP neuron | LP neuron | None | None | None | None |
28 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | 40 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | s-LNv neuron | LNd neuron | None | None | None | None |
4 | VFB_jrchjtf0 | DN1pA_R (FlyEM-HB:324846570) | 37 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | DN1p neuron | LNd neuron | None | None | None | None |
29 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | 37 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | LNd neuron | s-LNv neuron | None | None | None | None |
30 | VFB_jrchjtf2 | DN1pA_R (FlyEM-HB:387944118) | 34 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | DN1p neuron | LNd neuron | None | None | None | None |
21 | VFB_jrchjtf1 | DN1pA_R (FlyEM-HB:325529237) | 33 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | DN1p neuron | s-LNv neuron | None | None | None | None |
27 | VFB_jrchk08a | LPN_R (FlyEM-HB:450034902) | 30 | VFB_jrchk089 | LPN_R (FlyEM-HB:480029788) | LP neuron | LP neuron | None | None | None | None |
16 | VFB_jrchjtf1 | DN1pA_R (FlyEM-HB:325529237) | 30 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | DN1p neuron | LNd neuron | None | None | None | None |
9 | VFB_jrchjtf3 | DN1pA_R (FlyEM-HB:387166379) | 30 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | DN1p neuron | LNd neuron | None | None | None | None |
1 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | 29 | VFB_jrchjzxw | LNd_R (FlyEM-HB:5813056917) | LNd neuron | LNd neuron | None | None | None | None |
25 | VFB_jrchjtez | DN1pA_R (FlyEM-HB:5813010153) | 25 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | DN1p neuron | s-LNv neuron | None | None | None | None |
3 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | 25 | VFB_jrchjzxx | LNd_R (FlyEM-HB:5813021192) | s-LNv neuron | LNd neuron | None | None | None | None |
19 | VFB_jrchjtf0 | DN1pA_R (FlyEM-HB:324846570) | 25 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | DN1p neuron | s-LNv neuron | None | None | None | None |
2 | VFB_jrchjtf3 | DN1pA_R (FlyEM-HB:387166379) | 25 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | DN1p neuron | s-LNv neuron | None | None | None | None |
17 | VFB_jrchjtf2 | DN1pA_R (FlyEM-HB:387944118) | 22 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | DN1p neuron | s-LNv neuron | None | None | None | None |
22 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | 21 | VFB_jrchjzxx | LNd_R (FlyEM-HB:5813021192) | LNd neuron | LNd neuron | None | None | None | None |
8 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | 17 | VFB_jrchjzxw | LNd_R (FlyEM-HB:5813056917) | s-LNv neuron | LNd neuron | None | None | None | None |
12 | VFB_jrchjtez | DN1pA_R (FlyEM-HB:5813010153) | 15 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | DN1p neuron | LNd neuron | None | None | None | None |
23 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | 14 | VFB_jrchjtf3 | DN1pA_R (FlyEM-HB:387166379) | s-LNv neuron | DN1p neuron | None | None | None | None |
20 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | 14 | VFB_jrchjtf1 | DN1pA_R (FlyEM-HB:325529237) | LNd neuron | DN1p neuron | None | None | None | None |
13 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | 13 | VFB_jrchjtf3 | DN1pA_R (FlyEM-HB:387166379) | LNd neuron | DN1p neuron | None | None | None | None |
15 | VFB_jrchjtf0 | DN1pA_R (FlyEM-HB:324846570) | 13 | VFB_jrchjzxx | LNd_R (FlyEM-HB:5813021192) | DN1p neuron | LNd neuron | None | None | None | None |
18 | VFB_jrchk8e0 | 5th s-LNv (FlyEM-HB:511051477) | 12 | VFB_jrchjtf1 | DN1pA_R (FlyEM-HB:325529237) | s-LNv neuron | DN1p neuron | None | None | None | None |
24 | VFB_jrchjtf5 | DN1pB_R (FlyEM-HB:5813071319) | 11 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | DN1p neuron | LNd neuron | None | None | None | None |
6 | VFB_jrchjtey | DN1a_R (FlyEM-HB:5813022274) | 11 | VFB_jrchjtex | DN1a_R (FlyEM-HB:264083994) | DN1a neuron | DN1a neuron | None | None | None | None |
11 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | 10 | VFB_jrchjtez | DN1pA_R (FlyEM-HB:5813010153) | LNd neuron | DN1p neuron | None | None | None | None |
10 | VFB_jrchjtex | DN1a_R (FlyEM-HB:264083994) | 10 | VFB_jrchjtey | DN1a_R (FlyEM-HB:5813022274) | DN1a neuron | DN1a neuron | None | None | None | None |
5 | VFB_jrchjzxy | LNd_R (FlyEM-HB:5813069648) | 10 | VFB_jrchjtf2 | DN1pA_R (FlyEM-HB:387944118) | LNd neuron | DN1p neuron | None | None | None | None |
[ ]:
# Get connections between visual projectsion neurons and descending neurons
visPN2DC = vc.get_connected_neurons_by_type(upstream_type='visual projection neuron',
downstream_type='adult descending neuron',
weight=10).sort_values('weight', ascending=False)
visPN2DC
upstream_neuron_id | upstream_neuron_name | weight | downstream_neuron_id | downstream_neuron_name | upstream_class | downstream_class | up_data_source | up_accession | down_source | down_accession | |
---|---|---|---|---|---|---|---|---|---|---|---|
1060 | VFB_jrchk09j | LT51(aSP29a)_R (FlyEM-HB:1282348408) | 319 | VFB_jrchjtfr | DNb01_R (FlyEM-HB:1566597156) | lobula tangential neuron | descending neuron of the anterior ventral brai... | None | None | None | None |
293 | VFB_jrchk09c | LT51_R (FlyEM-HB:1444351896) | 221 | VFB_jrchjtfg | DNa03_R (FlyEM-HB:1139909038) | lobula tangential neuron | descending neuron of the anterior dorsal brain... | None | None | None | None |
403 | VFB_jrchk09j | LT51(aSP29a)_R (FlyEM-HB:1282348408) | 169 | VFB_jrchjthb | DNp18_R (FlyEM-HB:5813068840) | lobula tangential neuron | descending neuron of the posterior brain DNp18 | None | None | None | None |
143 | VFB_jrchk09i | LT51_R (FlyEM-HB:1597493900) | 141 | VFB_jrchjtfg | DNa03_R (FlyEM-HB:1139909038) | lobula tangential neuron | descending neuron of the anterior dorsal brain... | None | None | None | None |
483 | VFB_jrchk09l | LT51(aSP29a)_R (FlyEM-HB:1261229600) | 104 | VFB_jrchjtfr | DNb01_R (FlyEM-HB:1566597156) | lobula tangential neuron | descending neuron of the anterior ventral brai... | None | None | None | None |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
460 | VFB_jrchk0at | LT64_R (FlyEM-HB:1503042472) | 10 | VFB_jrchjtfl | DNa08(aSP22)_R (FlyEM-HB:1074782432) | lobula tangential neuron | descending neuron of the anterior dorsal brain... | None | None | None | None |
743 | VFB_jrchjzqr | LLPC2b (FlyEM-HB:5813024821) | 10 | VFB_jrchjtfy | DNb05_R (FlyEM-HB:1406966879) | lobula complex columnar neuron | descending neuron of the anterior ventral brai... | None | None | None | None |
464 | VFB_jrchjzrd | LLPC2b (FlyEM-HB:5812997920) | 10 | VFB_jrchjthq | DNp31_R (FlyEM-HB:1006984280) | lobula complex columnar neuron | descending neuron of the posterior brain DNp31 | None | None | None | None |
467 | VFB_jrchjy9c | LC4 (FlyEM-HB:1876902545) | 10 | VFB_jrchjtgf | DNp11_R (FlyEM-HB:1281324958) | lobula columnar neuron LC4 | descending neuron of the posterior brain DNp11 | None | None | None | None |
400 | VFB_jrchk05s | LPLC2_R (FlyEM-HB:1753674977) | 10 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) | lobula complex columnar neuron LPLC2 | descending neuron of the posterior brain DNp04 | None | None | None | None |
1085 rows × 11 columns
[ ]:
visPN2DC['upstream_class'].value_counts(normalize=True).plot(kind = 'bar') # Excercise - sum up weights for each type. Note - some types here subsume others
<AxesSubplot:>
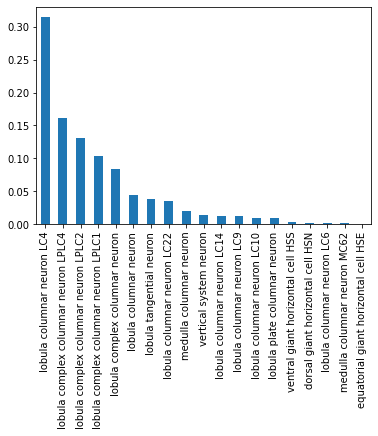
Get neurons downstream/downstream of a specific neuron
Starting from an individual neuron, in this case an individual neuron from hemibrain, find all neurons upstream or downstream, restricting to edges >= some specified wieght (synaptic connections) and optionally limiting the output by neuron type/class.
[ ]:
# Starting point - let's take one of the individual descending neurons from
# the previous exploratory queries and look for other inputs
upstream_of_DNp04 = vc.get_neurons_upstream_of('DNp04_R (FlyEM-HB:1405231475)', weight = 20)
upstream_of_DNp04
query_neuron_id | query_neuron_name | weight | target_neuron_id | target_neuron_name | |
---|---|---|---|---|---|
0 | VFB_jrchjy8y | LC4 (FlyEM-HB:1249932198) | 78 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
1 | VFB_jrchjy9n | LC4 (FlyEM-HB:1781268241) | 39 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
2 | VFB_jrchk4z0 | SAD017_R (FlyEM-HB:1630678915) | 41 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
3 | VFB_jrchjyap | LC4 (FlyEM-HB:1998922583) | 66 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
4 | VFB_jrchjyaj | LC4 (FlyEM-HB:1907578957) | 70 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
... | ... | ... | ... | ... | ... |
82 | VFB_jrchjyjy | LHAD1g1_R (FlyEM-HB:792326206) | 27 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
83 | VFB_jrchjy9d | LC4 (FlyEM-HB:1627117134) | 46 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
84 | VFB_jrchjy91 | LC4 (FlyEM-HB:1405780725) | 55 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
85 | VFB_jrchk4qn | PVLP046_R (FlyEM-HB:1600666632) | 48 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
86 | VFB_jrchjy9h | LC4 (FlyEM-HB:1688505620) | 43 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
87 rows × 5 columns
[ ]:
pd.DataFrame.from_records(vc.neo_query_wrapper.get_anatomical_individual_TermInfo(upstream_of_DNp04['query_neuron_id'], summary=True))
label | symbol | id | tags | parents_label | parents_id | data_source | accession | templates | dataset | license | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | LC4 (FlyEM-HB:1936848448) | VFB_jrchjyan | Entity|has_image|Adult|Anatomy|Glutamatergic|C... | lobula columnar neuron LC4 | FBbt_00003874 | neuprint_JRC_Hemibrain_1point1 | 1936848448 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
1 | LC4(lVLPT8)_R (FlyEM-HB:1907574944) | VFB_jrchjy8r | Entity|has_image|Adult|Anatomy|Glutamatergic|C... | lobula columnar neuron LC4 | FBbt_00003874 | neuprint_JRC_Hemibrain_1point1 | 1907574944 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
2 | LC4 (FlyEM-HB:1937875810) | VFB_jrchjya9 | Entity|has_image|Adult|Anatomy|Glutamatergic|C... | lobula columnar neuron LC4 | FBbt_00003874 | neuprint_JRC_Hemibrain_1point1 | 1937875810 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
3 | PVLP142_R (FlyEM-HB:1876565477) | VFB_jrchk4wk | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult posterior ventrolateral protocerebrum ne... | FBbt_20002200 | neuprint_JRC_Hemibrain_1point1 | 1876565477 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
4 | LC4 (FlyEM-HB:1878271377) | VFB_jrchjy9l | Entity|has_image|Adult|Anatomy|Glutamatergic|C... | lobula columnar neuron LC4 | FBbt_00003874 | neuprint_JRC_Hemibrain_1point1 | 1878271377 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
82 | LC4 (FlyEM-HB:1907924777) | VFB_jrchjy9r | Entity|has_image|Adult|Anatomy|Glutamatergic|C... | lobula columnar neuron LC4 | FBbt_00003874 | neuprint_JRC_Hemibrain_1point1 | 1907924777 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
83 | LC4 (FlyEM-HB:1625080038) | VFB_jrchjy9e | Entity|has_image|Adult|Anatomy|Glutamatergic|C... | lobula columnar neuron LC4 | FBbt_00003874 | neuprint_JRC_Hemibrain_1point1 | 1625080038 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
84 | SAD017_R (FlyEM-HB:1630678915) | VFB_jrchk4z0 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult saddle neuron 017 | FBbt_20002226 | neuprint_JRC_Hemibrain_1point1 | 1630678915 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
85 | LC4 (FlyEM-HB:1876471221) | VFB_jrchjya7 | Entity|has_image|Adult|Anatomy|Glutamatergic|C... | lobula columnar neuron LC4 | FBbt_00003874 | neuprint_JRC_Hemibrain_1point1 | 1876471221 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
86 | LC4 (FlyEM-HB:5813055129) | VFB_jrchjy9f | Entity|has_image|Adult|Anatomy|Glutamatergic|C... | lobula columnar neuron LC4 | FBbt_00003874 | neuprint_JRC_Hemibrain_1point1 | 5813055129 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None |
87 rows × 11 columns
Let’s try a similar query from one of the LC4 neurons to see what other descending neuron types are downstream of it.
[ ]:
vc.get_neurons_downstream_of('LC4 (FlyEM-HB:1249932198)', classification="'adult descending neuron'", weight = 10)
Running query: FBbt:00047511
Query URL: http://owl.virtualflybrain.org/kbs/vfb/instances?object=FBbt%3A00047511&prefixes=%7B%22FBbt%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FFBbt_%22%2C+%22RO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FRO_%22%2C+%22BFO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FBFO_%22%7D&direct=False
Query results: 142
query_neuron_id | query_neuron_name | weight | target_neuron_id | target_neuron_name | |
---|---|---|---|---|---|
0 | VFB_jrchjy8y | LC4 (FlyEM-HB:1249932198) | 78 | VFB_jrchjtg7 | DNp04_R (FlyEM-HB:1405231475) |
1 | VFB_jrchjy8y | LC4 (FlyEM-HB:1249932198) | 30 | VFB_jrchjup1 | Giant Fiber_R (FlyEM-HB:2307027729) |
2 | VFB_jrchjy8y | LC4 (FlyEM-HB:1249932198) | 65 | VFB_jrchjtgf | DNp11_R (FlyEM-HB:1281324958) |
3 | VFB_jrchjy8y | LC4 (FlyEM-HB:1249932198) | 11 | VFB_jrchjtg6 | DNp03_R (FlyEM-HB:1565846637) |
More sophisticated connectomics queries require direct queries of connectomics DB APIs
CATMAID connectivity queries
Broadly speaking, using pymaid to query CATMAID servers lets you fetch connectivity data as either lists of up- and downstream partners or as whole adjacency matrices.
These examples use the VFB FAFB server.
[ ]:
# Using VFB to get neurons by type
DA1 = vc.get_instances("'adult antennal lobe projection neuron DA1'", summary=True)
DA1_tab = pd.DataFrame.from_records(DA1)
len(DA1_tab)
DA1_tab
Running query: FBbt:00048096
Query URL: http://owl.virtualflybrain.org/kbs/vfb/instances?object=FBbt%3A00048096&prefixes=%7B%22FBbt%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FFBbt_%22%2C+%22RO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FRO_%22%2C+%22BFO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FBFO_%22%7D&direct=False
Query results: 26
label | symbol | id | tags | parents_label | parents_id | data_source | accession | templates | dataset | license | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | Uniglomerular mALT DA1 lPN#L7 (FAFB:3239781) | VFB_0010124l | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 3239781 | JRC2018Unisex | None | ||
1 | Uniglomerular mALT DA1 lPN#L5 (FAFB:2380564) | VFB_0010122z | Entity|has_image|Adult|Anatomy|Cell|Cholinergi... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 2380564 | JRC2018Unisex | None | ||
2 | Uniglomerular mALT DA1 lPN#L6 (FAFB:2381753) | VFB_0010123b | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 2381753 | JRC2018Unisex | None | ||
3 | Uniglomerular mALT DA1 lPN#R2 (FAFB:57311) | VFB_00101200 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 57311 | adult brain template JFRC2|JRC2018Unisex | None | ||
4 | DA1_lPN_R (FlyEM-HB:5813039315) | VFB_jrchjtdd | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | neuprint_JRC_Hemibrain_1point1 | 5813039315 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
5 | ALv1_P02(DA1)_0_2018U | VFB_00102294 | Entity|GABAergic|Adult|Anatomy|has_image|Cell|... | adult antennal lobe projection neuron DA1 vPN | FBbt_00067372 | JRC2018Unisex | None | ||||
6 | Uniglomerular mALT DA1 lPN#L2 (FAFB:2319457) | VFB_0010122k | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 2319457 | JRC2018Unisex | None | ||
7 | Uniglomerular mlALT DA1 vPN#L1 (FAFB:2334841) | VFB_0010122m | Entity|GABAergic|Adult|Anatomy|has_image|Cell|... | adult antennal lobe projection neuron DA1 vPN | FBbt_00067372 | catmaid_fafb | 2334841 | JRC2018Unisex | None | ||
8 | Uniglomerular mALT DA1 lPN#R5 (FAFB:2863104) | VFB_0010124e | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 2863104 | JRC2018Unisex | None | ||
9 | DA1_lPN_R (FlyEM-HB:1734350908) | VFB_jrchjtdb | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | neuprint_JRC_Hemibrain_1point1 | 1734350908 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
10 | DA1_lPN_R (FlyEM-HB:722817260) | VFB_jrchjtda | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | neuprint_JRC_Hemibrain_1point1 | 722817260 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
11 | Uniglomerular mALT DA1 lPN#L4 (FAFB:2379517) | VFB_0010122y | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 2379517 | JRC2018Unisex | None | ||
12 | Uniglomerular mALT DA1 lPN#R3 (FAFB:61221) | VFB_00101204 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 61221 | JRC2018Unisex|adult brain template JFRC2 | None | ||
13 | DA1_lPN_R (FlyEM-HB:754538881) | VFB_jrchjtdg | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | neuprint_JRC_Hemibrain_1point1 | 754538881 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
14 | Uniglomerular mALT DA1 lPN#R4 (FAFB:755022) | VFB_00101205 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 755022 | adult brain template JFRC2|JRC2018Unisex | None | ||
15 | Uniglomerular mALT DA1 lPN#R7 (FAFB:57353) | VFB_00101202 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 57353 | adult brain template JFRC2|JRC2018Unisex | None | ||
16 | Uniglomerular mALT DA1 lPN#L1 (FAFB:4207871) | VFB_0010126e | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 4207871 | JRC2018Unisex | None | ||
17 | DA1_lPN_R (FlyEM-HB:1765040289) | VFB_jrchjtdc | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | neuprint_JRC_Hemibrain_1point1 | 1765040289 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
18 | Uniglomerular mALT DA1 lPN#R6 (FAFB:27295) | VFB_00101199 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 27295 | adult brain template JFRC2|JRC2018Unisex | None | ||
19 | Uniglomerular mALT DA1 lPN#R1 (FAFB:57323) | VFB_00101201 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 57323 | adult brain template JFRC2|JRC2018Unisex | None | ||
20 | DA1_lPN_R (FlyEM-HB:754534424) | VFB_jrchjtde | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | neuprint_JRC_Hemibrain_1point1 | 754534424 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
21 | Uniglomerular mALT DA1 lPN#R8 (FAFB:57381) | VFB_00101203 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 57381 | JRC2018Unisex|adult brain template JFRC2 | None | ||
22 | Uniglomerular mALT DA1 lPN#L3 (FAFB:2345089) | VFB_0010122p | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | catmaid_fafb | 2345089 | JRC2018Unisex | None | ||
23 | Uniglomerular mlALT DA1 vPN#R1 (FAFB:1811442) | VFB_0010121x | Entity|GABAergic|Adult|Anatomy|has_image|Cell|... | adult antennal lobe projection neuron DA1 vPN | FBbt_00067372 | catmaid_fafb | 1811442 | JRC2018Unisex | None | ||
24 | DA1_lPN_R (FlyEM-HB:1734350788) | VFB_jrchjtdf | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | neuprint_JRC_Hemibrain_1point1 | 1734350788 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
25 | DA1_vPN_R (FlyEM-HB:733316908) | VFB_jrchjtdh | Entity|GABAergic|Adult|Anatomy|has_image|Cell|... | adult antennal lobe projection neuron DA1 vPN | FBbt_00067372 | neuprint_JRC_Hemibrain_1point1 | 733316908 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None |
[ ]:
# Map neurons to CATMAID Skeleton IDs (skids)
da1_skids = vc.neo_query_wrapper.vfb_id_2_xrefs(DA1_tab['id'], db='catmaid_fafb', reverse_return=True)
da1_skids_int = list(map(int, da1_skids))
da1_skids_int
/root/venv/lib/python3.7/site-packages/vfb_connect/neo/query_wrapper.py:308: UserWarning:
The following IDs do not match DB &/or id_type constraints: {'VFB_00101202', 'VFB_0010122p', 'VFB_jrchjtdb', 'VFB_00101199', 'VFB_jrchjtdf', 'VFB_jrchjtdg', 'VFB_jrchjtdd', 'VFB_0010122z', 'VFB_0010124l', 'VFB_0010122y', 'VFB_jrchjtda', 'VFB_00101201', 'VFB_jrchjtde', 'VFB_0010124e', 'VFB_0010122m', 'VFB_0010121x', 'VFB_00101205', 'VFB_0010126e', 'VFB_0010122k', 'VFB_00101203', 'VFB_00102294', 'VFB_jrchjtdc', 'VFB_00101200', 'VFB_00101204', 'VFB_jrchjtdh', 'VFB_0010123b'}
[2863104,
2381753,
57353,
1811442,
57323,
2345089,
57311,
27295,
2380564,
755022,
2379517,
61221,
57381,
4207871,
3239781,
2319457,
2334841]
Generate a connectivity table for neurons downstream of DA1 neurons
[ ]:
da1 = pymaid.get_neurons(da1_skids_int)
da1_ds = pymaid.get_partners(da1_skids_int,
threshold=3, # anything with >= 3 synapses
directions=['outgoing'] # downstream partners only
)
# Result is a pandas DataFrame
da1_ds.head()
INFO : Fetching connectivity table for 17 neurons (pymaid)
INFO : Done. Found 0 pre-, 270 postsynaptic and 0 gap junction-connected neurons (pymaid)
neuron_name | skeleton_id | num_nodes | relation | 2863104 | 2381753 | 57353 | 1811442 | 57323 | 2345089 | ... | 2380564 | 755022 | 2379517 | 61221 | 57381 | 4207871 | 3239781 | 2319457 | 2334841 | total | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | Uniglomerular mlALT DA1 vPN mlALTed Milk 18114... | 1811442 | 11769 | downstream | 30 | 0 | 3 | 0 | 20 | 0 | ... | 0 | 32 | 0 | 26 | 4 | 0 | 0 | 0 | 0 | 151.0 |
1 | Uniglomerular mlALT DA1 vPN mlALTed Milk 23348... | 2334841 | 6362 | downstream | 0 | 32 | 0 | 0 | 0 | 14 | ... | 0 | 0 | 28 | 0 | 0 | 17 | 26 | 22 | 0 | 139.0 |
2 | LHAV4a4#1 1911125 FML PS RJVR | 1911124 | 6969 | downstream | 23 | 0 | 6 | 0 | 15 | 0 | ... | 0 | 19 | 0 | 13 | 9 | 0 | 0 | 0 | 0 | 109.0 |
3 | LHAV2a3#1 1870231 RJVR AJES PS | 1870230 | 14820 | downstream | 5 | 0 | 23 | 1 | 7 | 0 | ... | 0 | 19 | 0 | 7 | 28 | 0 | 0 | 0 | 0 | 105.0 |
4 | LHAV4c1#1 488056 downstream DA1 GSXEJ | 488055 | 12137 | downstream | 15 | 0 | 3 | 0 | 11 | 0 | ... | 0 | 15 | 0 | 15 | 0 | 0 | 0 | 0 | 0 | 92.0 |
5 rows × 22 columns
[ ]:
# Get an adjacency matrix between all Bates, Schlegel et al. neurons
bates = pymaid.find_neurons(annotations='Paper: Bates and Schlegel et al 2020')
adj = pymaid.adjacency_matrix(bates)
adj.head()
INFO : Found 583 neurons matching the search parameters (pymaid)
targets | 2863104 | 57349 | 57353 | 16 | 57361 | 15738898 | 57365 | 4182038 | 3813399 | 11524119 | ... | 57323 | 4624362 | 1853423 | 2842610 | 57333 | 4624374 | 3080183 | 57337 | 4624378 | 57341 |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
sources | |||||||||||||||||||||
2863104 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 2.0 | 0.0 | 12.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 |
57349 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 |
57353 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0.0 | 0.0 | 5.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 |
16 | 0.0 | 0.0 | 0.0 | 1.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 1.0 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 |
57361 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | ... | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 | 0.0 |
5 rows × 583 columns
[ ]:
import seaborn as sns
ax = sns.clustermap(adj, vmax=10, cmap='Greys')
# ax = sns.heatmap(adj, vmax=10, cmap='Greys')
/shared-libs/python3.7/py/lib/python3.7/site-packages/seaborn/matrix.py:649: UserWarning:
Clustering large matrix with scipy. Installing `fastcluster` may give better performance.
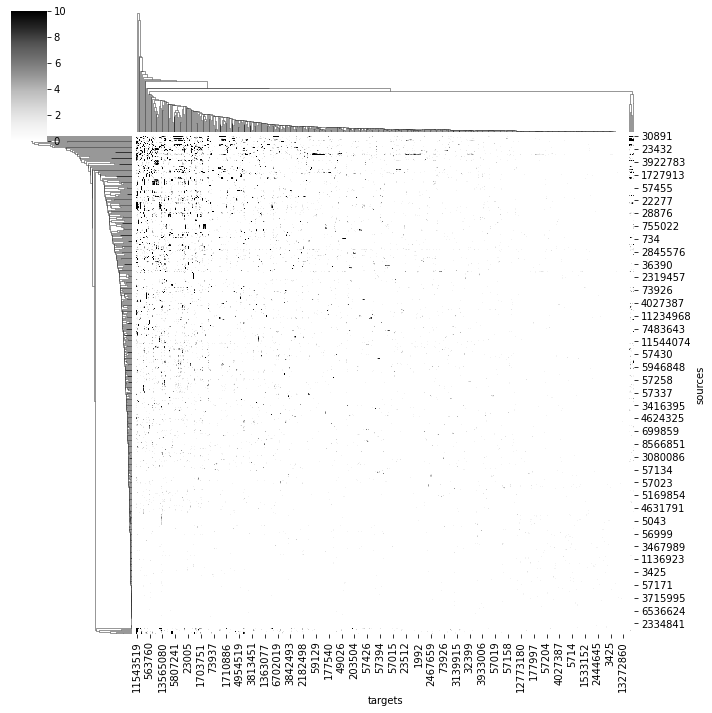
[ ]:
# Axo-axonic connections between two different types of DA1 PNs
cn = pymaid.get_connectors_between(2863104, 1811442)
cn.head()
connector_id | connector_loc | node1_id | source_neuron | confidence1 | creator1 | node1_loc | node2_id | target_neuron | confidence2 | creator2 | node2_loc | |
---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 6736296 | [359448.44, 159319.03, 150560.0] | 3163408 | 2863104 | 5 | NaN | [359487.3, 159145.66, 150600.0] | 6736298 | 1811442 | 5 | NaN | [359611.9, 159541.48, 150560.0] |
1 | 6795172 | [356041.88, 149555.53, 147920.0] | 6795195 | 2863104 | 5 | NaN | [354724.44, 149284.1, 147920.0] | 6795153 | 1811442 | 5 | NaN | [356366.16, 149854.86, 147920.0] |
2 | 6795291 | [355189.5, 150232.48, 148240.0] | 6795293 | 2863104 | 5 | NaN | [354595.62, 149464.8, 148240.0] | 6795214 | 1811442 | 5 | NaN | [355472.28, 150294.75, 148160.0] |
3 | 6795747 | [355030.4, 154047.86, 145800.0] | 6795749 | 2863104 | 5 | NaN | [355045.38, 154180.1, 145800.0] | 6795745 | 1811442 | 5 | NaN | [355024.44, 153945.73, 145760.0] |
4 | 6797452 | [353221.4, 148570.9, 147320.0] | 6797456 | 2863104 | 5 | NaN | [354213.9, 148397.44, 147320.0] | 6797437 | 1811442 | 5 | NaN | [353447.6, 148704.88, 147560.0] |
[ ]:
import numpy as np
points = np.vstack(cn.connector_loc)
navis.plot3d([da1.idx[[1811442, 57353]], # plot the two neurons
points], # plot the points of synaptic contacts as scatter
scatter_kws=dict(name="synaptic contacts")
)
neuprint connectivity queries
To programmatically interface with neuprint, we will use neuprint-python (link). It requires an API token which you can get via the website and is bound to the Google account that you use to log into neuprint. For this workshop we provide such a token as environment variable but you will need to start using your own token after the workshop is over.
These examples use the hemibrain v1.1 dataset.
neuprint-python
First we have to initialize the connection.
[ ]:
import neuprint as neu
client = neu.Client('https://neuprint.janelia.org',
dataset='hemibrain:v1.1',
token='eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJlbWFpbCI6InZmYndvcmtzaG9wLm5ldXJvZmx5MjAyMEBnbWFpbC5jb20iLCJsZXZlbCI6Im5vYXV0aCIsImltYWdlLXVybCI6Imh0dHBzOi8vbGg2Lmdvb2dsZXVzZXJjb250ZW50LmNvbS8tWXFDN21NRXd3TlEvQUFBQUFBQUFBQUkvQUFBQUFBQUFBQUEvQU1adXVjbU5zaXhXZDRhM0VyTTQ0ODBMa2IzNDdvUlpfUS9zOTYtYy9waG90by5qcGc_c3o9NTA_c3o9NTAiLCJleHAiOjE3OTQwOTE4ODd9.ceg4mrj2o-aOhK0NHNGmBacg8R34PBPoLBwhCo4uOCQ')
Most functions in neuprint-python
accept neu.NeuronCriteria
which is effectively a filter for body IDs, types, cellbody fibers (~lineages), etc:
[ ]:
help(neu.NeuronCriteria)
Help on class NeuronCriteria in module neuprint.neuroncriteria:
class NeuronCriteria(builtins.object)
| NeuronCriteria(matchvar='n', *, bodyId=None, instance=None, type=None, regex=False, cellBodyFiber=None, status=None, cropped=None, min_pre=0, min_post=0, rois=None, inputRois=None, outputRois=None, min_roi_inputs=1, min_roi_outputs=1, label=None, roi_req='all', client=None)
|
| Specifies which fields to filter by when searching for a Neuron (or Segment).
| This class does not send queries itself, but you use it to specify search
| criteria for various query functions.
|
| Note:
| For simple queries involving only particular bodyId(s) or type(s)/instance(s),
| you can usually just pass the ``bodyId`` or ``type`` to the query function,
| without constructing a full ``NeuronCriteria``.
|
| .. code-block:: python
|
| from neuprint import fetch_neurons, NeuronCriteria as NC
|
| # Equivalent
| neuron_df, conn_df = fetch_neurons(NC(bodyId=329566174))
| neuron_df, conn_df = fetch_neurons(329566174)
|
| # Equivalent
| # (Criteria is satisfied if either type or instance matches.)
| neuron_df, conn_df = fetch_neurons(NC(type="OA-VPM3", instance="OA-VPM3"))
| neuron_df, conn_df = fetch_neurons("OA-VPM3")
|
| Methods defined here:
|
| __eq__(self, value)
| Implement comparison between criteria.
| Note: 'matchvar' is not considered during the comparison.
|
| __init__(self, matchvar='n', *, bodyId=None, instance=None, type=None, regex=False, cellBodyFiber=None, status=None, cropped=None, min_pre=0, min_post=0, rois=None, inputRois=None, outputRois=None, min_roi_inputs=1, min_roi_outputs=1, label=None, roi_req='all', client=None)
| Except for ``matchvar``, all parameters must be passed as keyword arguments.
|
| .. note::
|
| **Options for specifying ROI criteria**
|
| The ``rois`` argument merely matches neurons that intersect the given ROIs at all
| (without distinguishing between inputs and outputs).
|
| The ``inputRois`` and ``outputRois`` arguments allow you to put requirements
| on whether or not neurons have inputs or outputs in the listed ROIs.
| It results a more expensive query, but its more powerful.
| It also enables you to require a minimum number of connections in the given
| ``inputRois`` or ``outputRois`` using the ``min_roi_inputs`` and ``min_roi_outputs``
| criteria.
|
| In either case, use use ``roi_req`` to specify whether a neuron must match just
| one (``any``) of the listed ROIs, or ``all`` of them.
|
| Args:
| matchvar (str):
| An arbitrary cypher variable name to use when this
| ``NeuronCriteria`` is used to construct cypher queries.
| To help catch errors (such as accidentally passing a ``type`` or
| ``instance`` name in the wrong argument position), we require that
| ``matchvar`` begin with a lowercase letter.
|
| bodyId (int or list of ints):
| List of bodyId values.
|
| instance (str or list of str):
| If ``regex=True``, then the instance will be matched as a regular expression.
| Otherwise, only exact matches are found. To search for neurons with no instance
| at all, use ``instance=[None]``. If both ``type`` and ``instance`` criteria are
| supplied, any neuron that matches EITHER criteria will match the overall criteria.
|
| type (str or list of str):
| If ``regex=True``, then the type will be matched as a regular expression.
| Otherwise, only exact matches are found. To search for neurons with no type
| at all, use ``type=[None]``. If both ``type`` and ``instance`` criteria are
| supplied, any neuron that matches EITHER criteria will match the overall criteria.
|
| regex (bool):
| If ``True``, the ``instance`` and ``type`` arguments will be interpreted as
| regular expressions, rather than exact match strings.
|
| cellBodyFiber (str or list of str):
| Matches for the neuron ``cellBodyFiber`` field. To search for neurons
| with no CBF at all, use ``cellBodyFiber=[None]``.
|
| status (str or list of str):
| Matches for the neuron ``status`` field. To search for neurons with no status
| at all, use ``status=[None]``.
|
| cropped (bool):
| If given, restrict results to neurons that are cropped or not.
|
| min_pre (int):
| Exclude neurons that don't have at least this many t-bars (outputs) overall,
| regardless of how many t-bars exist in any particular ROI.
|
| min_post (int):
| Exclude neurons that don't have at least this many PSDs (inputs) overall,
| regardless of how many PSDs exist in any particular ROI.
|
| rois (str or list of str):
| ROIs that merely intersect the neuron, without specifying whether
| they're intersected by input or output synapses.
| If not provided, will be auto-set from ``inputRois`` and ``outputRois``.
|
| inputRois (str or list of str):
| Only Neurons which have inputs in EVERY one of the given ROIs will be matched.
| ``regex`` does not apply to this parameter.
|
| outputRois (str or list of str):
| Only Neurons which have outputs in EVERY one of the given ROIs will be matched.
| ``regex`` does not apply to this parameter.
|
| min_roi_inputs (int):
| How many input (post) synapses a neuron must have in each ROI to satisfy the
| ``inputRois`` criteria. Can only be used if you provided ``inputRois``.
|
| min_roi_outputs (int):
| How many output (pre) synapses a neuron must have in each ROI to satisfy the
| ``outputRois`` criteria. Can only be used if you provided ``outputRois``.
|
| roi_req (Either ``'any'`` or ``'all'``):
| Whether a neuron must intersect all of the listed input/output ROIs, or any of the listed input/output ROIs.
| When using 'any', each neuron must still match at least one input AND at least one output ROI.
|
| label (Either ``'Neuron'`` or ``'Segment'``):
| Which node label to match with.
| (In neuprint, all ``Neuron`` nodes are also ``Segment`` nodes.)
| By default, ``'Neuron'`` is used, unless you provided a non-empty ``bodyId`` list.
| In that case, ``'Segment'`` is the default. (It's assumed you're really interested
| in the bodies you explicitly listed, whether or not they have the ``'Neuron'`` label.)
|
| client (:py:class:`neuprint.client.Client`):
| Used to validate ROI names.
| If not provided, the global default ``Client`` will be used.
|
| __repr__(self)
| Return repr(self).
|
| all_conditions(self, *vars, prefix=0, comments=True)
|
| basic_conditions(self, prefix=0, comments=True)
| Construct a WHERE clause based on the basic conditions
| in this criteria (i.e. everything except for the "directed ROI" conditions.)
|
| basic_exprs(self)
| Return the list of expressions that correspond
| to the members in this NeuronCriteria object.
| They're intended be combined (via 'AND') in
| the WHERE clause of a cypher query.
|
| bodyId_expr(self)
|
| cbf_expr(self)
|
| cropped_expr(self)
|
| directed_rois_condition(self, *vars, prefix=0, comments=True)
| Construct the ```WITH...WHERE``` statements that apply the "directed ROI"
| conditions specified by this criteria's ``inputRois`` and ``outputRois``
| members.
|
| These conditions are expensive to evaluate, so it's usually a good
| idea to position them LAST in your cypher query, once the result set
| has already been narrowed down by eariler filters.
|
| global_vars(self)
|
| global_with(self, *vars, prefix=0)
|
| instance_expr(self)
|
| post_expr(self)
|
| pre_expr(self)
|
| rois_expr(self)
|
| status_expr(self)
|
| type_expr(self)
|
| typeinst_expr(self)
| Unlike all other fields, type and instance OR'd together.
| Either match satisfies the criteria.
|
| ----------------------------------------------------------------------
| Class methods defined here:
|
| combined_conditions(neuron_conditions, vars=[], prefix=0, comments=True) from builtins.type
| Combine the conditions from multiple NeuronCriteria into a single string,
| putting the "cheap" conditions first and the "expensive" conditions last.
| (That is, basic conditions first and the directed ROI conditions last.)
|
| combined_global_with(neuron_conditions, vars=[], prefix=0) from builtins.type
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| MAX_LITERAL_LENGTH = 3
|
| __hash__ = None
Fetching neurons
Let’s say we want to find all antennnal lobe projection neurons (PNs). Their type nomenclature adheres to {glomerulus}_{lineage}PN
(e.g. DA1_lPN
)for uniglomerular PNs and a M_{lineage}PN{tract}{type}
(e.g. M_vPNml50
= “multiglomerular ventral lineage PN mediolateral tract type 50) for multiglomerular PNs.
To get them all, we need to use regex patterns (see this cheatsheet):
[ ]:
# Define the filter criteria
nc = neu.NeuronCriteria(type='.*?_.*?PN.*?', regex=True)
# Get general info for these neurons
pns, roi_info = neu.fetch_neurons(nc)
print(f'{pns.shape[0]} PNs found.')
pns.head()
337 PNs found.
bodyId | instance | type | pre | post | downstream | upstream | mito | size | status | cropped | statusLabel | cellBodyFiber | somaRadius | somaLocation | inputRois | outputRois | roiInfo | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 294792184 | M_vPNml53_R | M_vPNml53 | 92 | 344 | 730 | 344 | None | 420662445 | Traced | False | Roughly traced | AVM04 | 336.5 | [18923, 34319, 35424] | [AL(R), AL-D(R), AL-DA2(R), AL-DA4m(R), AL-DC1... | [AL(R), AL-DC1(R), LH(R), PLP(R), SIP(R), SLP(... | {'SNP(R)': {'pre': 70, 'post': 155, 'downstrea... |
1 | 329599710 | M_lvPNm32_R | M_lvPNm32 | 247 | 285 | 2052 | 285 | None | 343478957 | Traced | False | Roughly traced | AVM06 | NaN | None | [AL(R), AL-DC4(R), AL-DL2v(R), AL-DM1(R), AL-D... | [AL(R), AL-DL2v(R), AL-DM1(R), AL-DM4(R), AL-D... | {'SNP(R)': {'pre': 180, 'post': 93, 'downstrea... |
2 | 417199910 | M_lvPNm36_R | M_lvPNm36 | 162 | 347 | 1590 | 347 | None | 387058559 | Traced | False | Roughly traced | AVM06 | 351.5 | [13823, 33925, 34176] | [AL(R), AL-DL5(R), AL-DM4(R), AL-DP1m(R), AL-V... | [AL(R), AL-DL5(R), AL-DM4(R), AL-VP1d(R), AL-V... | {'SNP(R)': {'pre': 156, 'post': 95, 'downstrea... |
3 | 480927537 | M_vPNml70_R | M_vPNml70 | 82 | 276 | 692 | 276 | None | 240153322 | Traced | False | Roughly traced | AVM04 | NaN | None | [AL(R), AL-DA2(R), AL-DA4l(R), AL-DA4m(R), AL-... | [LH(R), SLP(R), SNP(R)] | {'SNP(R)': {'pre': 15, 'post': 18, 'downstream... |
4 | 481268653 | M_vPNml89_R | M_vPNml89 | 146 | 58 | 1614 | 58 | None | 265085609 | Traced | False | Roughly traced | AVM04 | NaN | None | [AL(R), AL-VC3l(R), AL-VC4(R), AL-VP1m(R), LH(... | [LH(R), SLP(R), SNP(R)] | {'SNP(R)': {'pre': 10, 'post': 2, 'downstream'... |
[ ]:
# Check that the regex did not have any accidental by-catch
pns['type'].unique()
array(['M_vPNml53', 'M_lvPNm32', 'M_lvPNm36', 'M_vPNml70', 'M_vPNml89',
'VP1l+_lvPN', 'M_vPNml69', 'DM1_lPN', 'DM4_vPN', 'M_vPNml79',
'VP4+_vPN', 'DA4l_adPN', 'M_vPNml87', 'DM4_adPN', 'M_vPNml83',
'VA5_lPN', 'DA4m_adPN', 'M_lvPNm24', 'M_vPNml85', 'VP1l+VP3_ilPN',
'M_vPNml77', 'M_vPNml84', 'VC1_lPN', 'M_lvPNm39', 'M_vPNml50',
'DM2_lPN', 'VC5_lvPN', 'M_vPNml88', 'M_vPNml58', 'VP4_vPN',
'DP1m_vPN', 'DP1m_adPN', 'DM5_lPN', 'VC5_adPN', 'M_vPNml80',
'M_lvPNm25', 'VC3m_lvPN', 'VP3+_vPN', 'VP1m+_lvPN', 'DA3_adPN',
'V_l2PN', 'M_vPNml56', 'VC3l_adPN', 'VM7v_adPN', 'DL5_adPN',
'VM4_adPN', 'VM2_adPN', 'M_lvPNm40', 'DC4_vPN', 'V_ilPN',
'M_vPNml74', 'Z_lvPNm1', 'DA1_lPN', 'DP1l_adPN', 'VM4_lvPN',
'M_vPNml71', 'DP1l_vPN', 'M_lvPNm41', 'M_spPN5t10', 'DA1_vPN',
'VC4_adPN', 'DM3_adPN', 'M_lvPNm45', 'VL1_vPN', 'M_lvPNm44',
'M_vPNml78', 'M_vPNml67', 'M_adPNm5', 'M_smPNm1', 'DM6_adPN',
'DL2d_adPN', 'M_adPNm6', 'M_adPNm8', 'M_lvPNm43', 'Z_vPNml1',
'M_vPNml59', 'DA2_lPN', 'M_lPNm11A', 'M_vPNml52', 'DL2d_vPN',
'VL2p_vPN', 'VA1d_adPN', 'M_lPNm11B', 'M_lvPNm48', 'M_lPNm11C',
'M_lvPNm42', 'VA1v_vPN', 'M_vPNml68', 'M_vPNml55', 'M_vPNml62',
'VL2a_vPN', 'M_vPNml60', 'M_vPNml65', 'VM5d_adPN', 'M_l2PNm16',
'M_vPNml61', 'M_vPNml57', 'M_vPNml64', 'M_lv2PN9t49',
'VP2+VC5_l2PN', 'M_spPN4t9', 'M_vPNml66', 'M_vPNml75', 'M_vPNml63',
'M_vPNml72', 'M_lvPNm38', 'D_adPN', 'M_vPNml76', 'M_vPNml54',
'DM3_vPN', 'M_vPNml86', 'DL3_lPN', 'VA4_lPN', 'VP1d_il2PN',
'DC1_adPN', 'M_l2PN3t18', 'M_lvPNm35', 'DL4_adPN', 'M_lvPNm28',
'M_lvPNm27', 'M_ilPNm90', 'M_l2PNl20', 'M_lvPNm29', 'VA7l_adPN',
'M_lPNm13', 'M_l2PNl21', 'DL1_adPN', 'M_imPNl92', 'M_vPNml73',
'M_ilPN8t91', 'M_l2PNm14', 'VP1d+VP4_l2PN1', 'M_lvPNm26',
'DL2v_adPN', 'VP3+VP1l_ivPN', 'M_lvPNm33', 'VA1v_adPN',
'VP3+_l2PN', 'M_l2PN10t19', 'VP4+VL1_l2PN', 'M_l2PNl22',
'M_l2PNm15', 'M_lPNm11D', 'MZ_lv2PN', 'DC2_adPN', 'M_lvPNm46',
'VC2_lPN', 'VM1_lPN', 'VM3_adPN', 'VM7d_adPN', 'M_lvPNm47',
'M_lPNm12', 'DC3_adPN', 'VP2+_adPN', 'VP1m+VP2_lvPN2',
'VP1m+VP2_lvPN1', 'VA6_adPN', 'VA7m_lPN', 'M_adPNm7', 'M_adPNm4',
'VA1d_vPN', 'VA3_adPN', 'VL1_ilPN', 'M_l2PNl23', 'M_lvPNm31',
'VP1m+VP5_ilPN', 'VL2p_adPN', 'MZ_lvPN', 'VP2_adPN', 'VA2_adPN',
'VM5v_adPN', 'VP5+VP2_l2PN', 'VP5+VP3_l2PN', 'VP5+_l2PN',
'M_vPNml51', 'M_smPN6t2', 'M_lvPNm37', 'M_vPNml82', 'M_adPNm3',
'VP1m_l2PN', 'DC4_adPN', 'VP5+Z_adPN', 'VL2a_adPN', 'VP2_l2PN',
'M_lvPNm34', 'VP2+Z_lvPN', 'M_lvPNm30', 'M_l2PNm17', 'M_vPNml81',
'VP1d+VP4_l2PN2'], dtype=object)
We can find the same neuron types using VFB instead. This allows curated neuron classes to be used and doesn’t depend on knowing naming conventions and using potentially error prone regex pattern matching.
[ ]:
#This will get all ALPNs from ALL datasets on VFB
ALPNs = vc.get_instances("'adult antennal lobe projection neuron'", summary=True)
ALPNs = pd.DataFrame.from_records(ALPNs)
#Select only rows from Hemibrain1.1 dataset
ALPNs=ALPNs[ALPNs['data_source'].str.match('neuprint_JRC_Hemibrain_1point1')]
ALPNs
Running query: FBbt:00067123
Query URL: http://owl.virtualflybrain.org/kbs/vfb/instances?object=FBbt%3A00067123&prefixes=%7B%22FBbt%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FFBbt_%22%2C+%22RO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FRO_%22%2C+%22BFO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FBFO_%22%7D&direct=False
Query results: 1659
label | symbol | id | tags | parents_label | parents_id | data_source | accession | templates | dataset | license | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | M_adPNm4_R (FlyEM-HB:1887872301) | VFB_jrchk0vj | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult multiglomerular antennal lobe projection... | FBbt_20003790 | neuprint_JRC_Hemibrain_1point1 | 1887872301 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
4 | DA2_lPN_R (FlyEM-HB:1797505019) | VFB_jrchjtdk | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA2 lPN | FBbt_00110882 | neuprint_JRC_Hemibrain_1point1 | 1797505019 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
9 | M_lvPNm26_R (FlyEM-HB:5812995328) | VFB_jrchk0wt | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult multiglomerular antennal lobe projection... | FBbt_20003807 | neuprint_JRC_Hemibrain_1point1 | 5812995328 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
15 | M_vPNml83_R (FlyEM-HB:697145036) | VFB_jrchk0zn | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult multiglomerular antennal lobe projection... | FBbt_20003858 | neuprint_JRC_Hemibrain_1point1 | 697145036 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
21 | M_vPNml78_R (FlyEM-HB:760250511) | VFB_jrchk0zg | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult multiglomerular antennal lobe projection... | FBbt_20003853 | neuprint_JRC_Hemibrain_1point1 | 760250511 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
1628 | VC5_lvPN_R (FlyEM-HB:5812995098) | VFB_jrchk7dz | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult uniglomerular antennal lobe projection n... | FBbt_00007440 | neuprint_JRC_Hemibrain_1point1 | 5812995098 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
1634 | DA1_lPN_R (FlyEM-HB:722817260) | VFB_jrchjtda | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron DA1 lPN | FBbt_00067363 | neuprint_JRC_Hemibrain_1point1 | 722817260 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
1635 | M_lvPNm32_R (FlyEM-HB:329599710) | VFB_jrchk0x0 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult multiglomerular antennal lobe projection... | FBbt_20003813 | neuprint_JRC_Hemibrain_1point1 | 329599710 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
1638 | M_vPNml60_R (FlyEM-HB:975457916) | VFB_jrchk0yf | Entity|GABAergic|Adult|Anatomy|has_image|Cell|... | adult antennal lobe projection neuron VP1d++ vPN | FBbt_00049748 | neuprint_JRC_Hemibrain_1point1 | 975457916 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
1657 | M_lPNm11B_R (FlyEM-HB:850703925) | VFB_jrchk0wc | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult antennal lobe projection neuron VP1l++ lPN | FBbt_00049754 | neuprint_JRC_Hemibrain_1point1 | 850703925 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None |
339 rows × 11 columns
Fetching synaptic partners
Looks good! Next: What’s downstream of those PNs?
[ ]:
ds = neu.fetch_simple_connections(upstream_criteria=list(map(int, ALPNs['accession'])))
#using hemibrain regex
#ds = neu.fetch_simple_connections(upstream_criteria=neu.NeuronCriteria(bodyId=pns.bodyId.values))
ds
bodyId_pre | bodyId_post | weight | type_pre | type_post | instance_pre | instance_post | conn_roiInfo | |
---|---|---|---|---|---|---|---|---|
0 | 635062078 | 1671292719 | 390 | DP1m_adPN | lLN2T_c | DP1m_adPN_R | lLN2T_c(Tortuous)_R | {'AL(R)': {'pre': 390, 'post': 390}, 'AL-DP1m(... |
1 | 635062078 | 1704347707 | 326 | DP1m_adPN | lLN2T_c | DP1m_adPN_R | lLN2T_c(Tortuous)_R | {'AL(R)': {'pre': 324, 'post': 324}, 'AL-DP1m(... |
2 | 542634818 | 1704347707 | 322 | DM1_lPN | lLN2T_c | DM1_lPN_R | lLN2T_c(Tortuous)_R | {'AL(R)': {'pre': 322, 'post': 322}, 'AL-DM1(R... |
3 | 635062078 | 1640922516 | 320 | DP1m_adPN | lLN2T_e | DP1m_adPN_R | lLN2T_e(Tortuous)_R | {'AL(R)': {'pre': 317, 'post': 316}, 'AL-DP1m(... |
4 | 724816115 | 1670916819 | 318 | DP1l_adPN | lLN2P_a | DP1l_adPN_R | lLN2P_a(Patchy)_R | {'AL(R)': {'pre': 318, 'post': 318}, 'AL-DP1l(... |
... | ... | ... | ... | ... | ... | ... | ... | ... |
101862 | 5901222910 | 5813086037 | 1 | DM2_lPN | None | DM2_lPN_R | None | {'LH(R)': {'pre': 1, 'post': 1}} |
101863 | 5901222910 | 5813095915 | 1 | DM2_lPN | KCg-m | DM2_lPN_R | KCg-m_R | {'MB(R)': {'pre': 1, 'post': 1}, 'CA(R)': {'pr... |
101864 | 5901222910 | 5813129316 | 1 | DM2_lPN | LHAV6a1_b | DM2_lPN_R | LHAV6a1_b_R | {'LH(R)': {'pre': 1, 'post': 1}} |
101865 | 5901222910 | 5901193783 | 1 | DM2_lPN | LHAV4g4_a | DM2_lPN_R | LHAV4g4_a_R | {'LH(R)': {'pre': 1, 'post': 1}} |
101866 | 5901222910 | 5901203780 | 1 | DM2_lPN | LHAV4g11 | DM2_lPN_R | LHAV4g11_R | {'LH(R)': {'pre': 1, 'post': 1}} |
101867 rows × 8 columns
Each row is now a connections from a single up- to a single downstream neuron. The “weight” is the number of synapses between the pre- and the postsynaptic neuron. Let’s simplify by grouping by type:
[ ]:
by_type = ds.groupby(['type_pre', 'type_post'], as_index=False).weight.sum()
by_type.sort_values('weight', ascending=False, inplace=True)
by_type.reset_index(drop=True, inplace=True)
by_type
type_pre | type_post | weight | |
---|---|---|---|
0 | DC3_adPN | KCg-m | 3670 |
1 | VM5d_adPN | KCg-m | 3219 |
2 | DC1_adPN | KCg-m | 3215 |
3 | VL2a_adPN | KCg-m | 3096 |
4 | DA1_lPN | KCg-m | 3078 |
... | ... | ... | ... |
40631 | M_vPNml50 | WEDPN4 | 1 |
40632 | M_vPNml50 | WEDPN12 | 1 |
40633 | M_vPNml50 | V_ilPN | 1 |
40634 | M_vPNml50 | VP4+VL1_l2PN | 1 |
40635 | Z_vPNml1 | mALD2 | 1 |
40636 rows × 3 columns
The strongest connections are between PNs and Kenyon Cells (KCs). That’s not much of a surprise since there are thousands of KCs. For the sake of the argument let’s say we want to know where these connections occur:
[ ]:
#First find KCs in VFB
KCs = pd.DataFrame.from_records(vc.get_instances("'adult Kenyon cell'", summary=True))
#Select only rows from Hemibrain1.1 dataset
KCs=KCs[KCs['data_source'].str.match('neuprint_JRC_Hemibrain_1point1')]
KCs
Running query: FBbt:00049825
Query URL: http://owl.virtualflybrain.org/kbs/vfb/instances?object=FBbt%3A00049825&prefixes=%7B%22FBbt%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FFBbt_%22%2C+%22RO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FRO_%22%2C+%22BFO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FBFO_%22%7D&direct=False
Query results: 5110
label | symbol | id | tags | parents_label | parents_id | data_source | accession | templates | dataset | license | |
---|---|---|---|---|---|---|---|---|---|---|---|
1 | KCab-c_R (FlyEM-HB:5812983309) | VFB_jrchjvga | Entity|has_image|Adult|Anatomy|has_neuron_conn... | alpha/beta core Kenyon cell | FBbt_00110929 | neuprint_JRC_Hemibrain_1point1 | 5812983309 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
2 | KCab-c_R (FlyEM-HB:815926346) | VFB_jrchjvgn | Entity|has_image|Adult|Anatomy|has_neuron_conn... | alpha/beta core Kenyon cell | FBbt_00110929 | neuprint_JRC_Hemibrain_1point1 | 815926346 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
7 | KCa\'b\'-m_R (FlyEM-HB:861630613) | VFB_jrchjv8j | Entity|has_image|Adult|Anatomy|has_neuron_conn... | alpha'/beta' middle Kenyon cell | FBbt_00100253 | neuprint_JRC_Hemibrain_1point1 | 861630613 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
8 | KCg-m_R (FlyEM-HB:1173054679) | VFB_jrchjw29 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | gamma main Kenyon cell | FBbt_00111061 | neuprint_JRC_Hemibrain_1point1 | 1173054679 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
9 | KCab-m_R (FlyEM-HB:363384090) | VFB_jrchjvny | Entity|has_image|Adult|Anatomy|has_neuron_conn... | alpha/beta Kenyon cell | FBbt_00100248 | neuprint_JRC_Hemibrain_1point1 | 363384090 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
5102 | KCg-m_R (FlyEM-HB:5813081174) | VFB_jrchjwcs | Entity|has_image|Adult|Anatomy|has_neuron_conn... | gamma main Kenyon cell | FBbt_00111061 | neuprint_JRC_Hemibrain_1point1 | 5813081174 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
5103 | KCg-m_R (FlyEM-HB:1100675450) | VFB_jrchjwcr | Entity|has_image|Adult|Anatomy|has_neuron_conn... | gamma main Kenyon cell | FBbt_00111061 | neuprint_JRC_Hemibrain_1point1 | 1100675450 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
5104 | KCg-m_R (FlyEM-HB:5812981951) | VFB_jrchjwe4 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | gamma main Kenyon cell | FBbt_00111061 | neuprint_JRC_Hemibrain_1point1 | 5812981951 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
5105 | KCg-m_R (FlyEM-HB:880383629) | VFB_jrchjwe5 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | gamma main Kenyon cell | FBbt_00111061 | neuprint_JRC_Hemibrain_1point1 | 880383629 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
5106 | KCg-m_R (FlyEM-HB:694524222) | VFB_jrchjwdk | Entity|has_image|Adult|Anatomy|has_neuron_conn... | gamma main Kenyon cell | FBbt_00111061 | neuprint_JRC_Hemibrain_1point1 | 694524222 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None |
1927 rows × 11 columns
[ ]:
adj, roi_info2 = neu.fetch_adjacencies(sources=list(map(int, ALPNs['accession'][:300])), targets=list(map(int, KCs['accession'][:300])))
roi_info2.head()
bodyId_pre | bodyId_post | roi | weight | |
---|---|---|---|---|
0 | 542634818 | 332344908 | CA(R) | 9 |
1 | 542634818 | 332353106 | CA(R) | 13 |
2 | 542634818 | 332685751 | CA(R) | 12 |
3 | 542634818 | 363038831 | CA(R) | 30 |
4 | 542634818 | 363384138 | CA(R) | 29 |
[ ]:
# Group by region of interest (ROI)
by_roi = roi_info2.groupby('roi').weight.sum()
by_roi.head()
roi
CA(R) 22134
NotPrimary 234
SCL(R) 16
SLP(R) 364
Name: weight, dtype: int64
[ ]:
ax = by_roi.plot.bar()
ax.set_xlabel('')
ax.set_ylabel('PN to KC synapses')
Text(0, 0.5, 'PN to KC synapses')
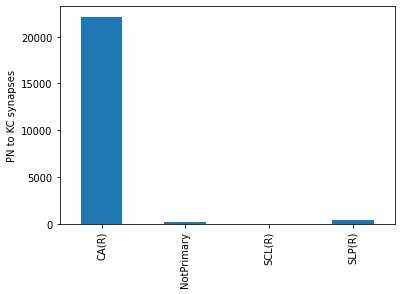
[ ]:
adj = roi_info2[roi_info2.roi == 'CA(R)'].pivot(columns="bodyId_pre",
index="bodyId_post",
values="weight").fillna(0)
[ ]:
sns.clustermap(adj, cmap='Greys')
/shared-libs/python3.7/py/lib/python3.7/site-packages/seaborn/matrix.py:649: UserWarning:
Clustering large matrix with scipy. Installing `fastcluster` may give better performance.
<seaborn.matrix.ClusterGrid at 0x7f4bd9c464d0>
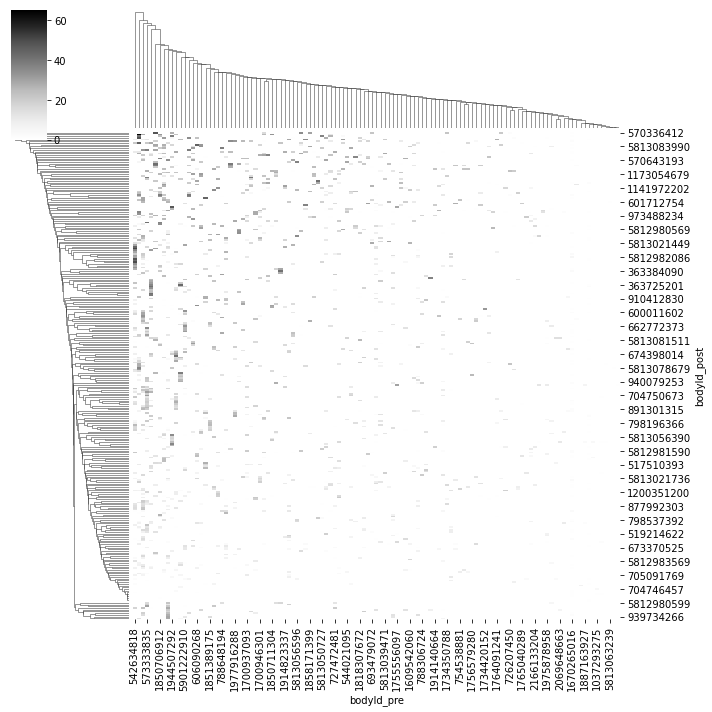
Querying paths
Let’s say we want to find out how to go from a PN (second order olfactory neurons) all the way to a descending neuron (presumably leading to motor neurons in the VNC).
[ ]:
#First find DNs in VFB
DNs = pd.DataFrame.from_records(vc.get_instances("'adult descending neuron'", summary=True))
#Select only rows from Hemibrain1.1 dataset
DNs=DNs[DNs['data_source'].str.match('neuprint_JRC_Hemibrain_1point1')]
DNs
Running query: FBbt:00047511
Query URL: http://owl.virtualflybrain.org/kbs/vfb/instances?object=FBbt%3A00047511&prefixes=%7B%22FBbt%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FFBbt_%22%2C+%22RO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FRO_%22%2C+%22BFO%22%3A+%22http%3A%2F%2Fpurl.obolibrary.org%2Fobo%2FBFO_%22%7D&direct=False
Query results: 142
label | symbol | id | tags | parents_label | parents_id | data_source | accession | templates | dataset | license | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | MDN_R (FlyEM-HB:5813021075) | VFB_jrchk0vd | Entity|has_image|Adult|Anatomy|has_neuron_conn... | adult moonwalker descending neuron | FBbt_00111308 | neuprint_JRC_Hemibrain_1point1 | 5813021075 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
1 | DNp16/17_R (FlyEM-HB:5813022707) | VFB_jrchjtgw | Entity|has_image|Adult|Anatomy|has_neuron_conn... | descending neuron of the posterior brain | FBbt_00047517 | neuprint_JRC_Hemibrain_1point1 | 5813022707 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
3 | Giant Fiber_R (FlyEM-HB:2307027729) | VFB_jrchjup1 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | giant fiber neuron | FBbt_00004020 | neuprint_JRC_Hemibrain_1point1 | 2307027729 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
4 | DNp06_R (FlyEM-HB:5813023322) | VFB_jrchjtg9 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | descending neuron of the posterior brain DNp06 | FBbt_00047639 | neuprint_JRC_Hemibrain_1point1 | 5813023322 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
5 | DNp17_R (FlyEM-HB:1654940174) | VFB_jrchjth5 | Entity|has_image|Adult|Anatomy|has_neuron_conn... | descending neuron of the posterior brain DNp17 | FBbt_00047650 | neuprint_JRC_Hemibrain_1point1 | 1654940174 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
136 | DNp15_R (FlyEM-HB:5813078134) | VFB_jrchjtgi | Entity|has_image|Adult|Anatomy|has_neuron_conn... | descending neuron of the posterior brain DNp15 | FBbt_00047648 | neuprint_JRC_Hemibrain_1point1 | 5813078134 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
137 | DNp16_R (FlyEM-HB:1436330964) | VFB_jrchjtgt | Entity|has_image|Adult|Anatomy|has_neuron_conn... | descending neuron of the posterior brain DNp16 | FBbt_00047649 | neuprint_JRC_Hemibrain_1point1 | 1436330964 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
139 | DNb01_R (FlyEM-HB:1655997973) | VFB_jrchjtfq | Entity|has_image|Adult|Anatomy|has_neuron_conn... | descending neuron of the anterior ventral brai... | FBbt_00047582 | neuprint_JRC_Hemibrain_1point1 | 1655997973 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None | ||
140 | DNp44_R (FlyEM-HB:542751938) | VFB_jrchjthu | Entity|has_image|Adult|Anatomy|has_neuron_conn... | descending neuron of the posterior brain DNp44 | FBbt_00049697 | neuprint_JRC_Hemibrain_1point1 | 542751938 | JRC_FlyEM_Hemibrain|JRC2018Unisex | None | ||
141 | DNp16_R (FlyEM-HB:1405300499) | VFB_jrchjtgm | Entity|has_image|Adult|Anatomy|has_neuron_conn... | descending neuron of the posterior brain DNp16 | FBbt_00047649 | neuprint_JRC_Hemibrain_1point1 | 1405300499 | JRC2018Unisex|JRC_FlyEM_Hemibrain | None |
101 rows × 11 columns
[ ]:
# First fetch the DNs
dns, _ = neu.fetch_neurons(list(map(int, DNs['accession'])))
#with regex
#dns, _ = neu.fetch_neurons(neu.NeuronCriteria(type='(.*DN[^1]{0,}.*|Giant Fiber)', regex=True))
dns
bodyId | instance | type | pre | post | downstream | upstream | mito | size | status | cropped | statusLabel | cellBodyFiber | somaRadius | somaLocation | inputRois | outputRois | roiInfo | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 451689001 | DNp25_R | DNp25 | 165 | 803 | 852 | 803 | None | 948309595 | Traced | False | Roughly traced | PDM05 | 361.0 | [22185, 13374, 16352] | [ATL(R), CA(R), FLA(R), INP, LH(R), MB(+ACA)(R... | [ATL(R), CA(R), INP, MB(+ACA)(R), MB(R), SLP(R... | {'SNP(R)': {'pre': 158, 'post': 703, 'downstre... |
1 | 512851433 | DNp10(PDM27)_L | DNp10 | 25 | 2487 | 121 | 2487 | None | 3010901036 | Traced | False | Roughly traced | None | 483.5 | [27334, 21053, 7640] | [ATL(L), GC, GOR(L), IB, ICL(L), INP, SCL(L), ... | [ATL(L), GC, GOR(L), ICL(L), INP, SCL(L), SMP(... | {'SNP(L)': {'pre': 7, 'post': 512, 'downstream... |
2 | 519949044 | oviDNb_R | oviDNb | 7 | 1089 | 21 | 1089 | None | 1618057638 | Traced | False | Roughly traced | PDM15 | 396.0 | [19291, 15307, 11696] | [AVLP(R), CA(R), CRE(-ROB,-RUB)(R), CRE(-RUB)(... | [CRE(-RUB)(L), CRE(L), INP, SIP(R), SMP(L), SM... | {'SNP(R)': {'pre': 2, 'post': 656, 'downstream... |
3 | 542751938 | DNp44_R | DNp44 | 182 | 634 | 978 | 634 | None | 1674610618 | Traced | False | Roughly traced | PVM09 | 321.0 | [18875, 11698, 13136] | [AL(R), AL-VL1(R), AL-VL2p(R), AL-VP1d(R), AL-... | [AL(R), AL-DP1m(R), AL-VL1(R), AL-VL2p(R), AL-... | {'SNP(R)': {'pre': 21, 'post': 277, 'downstrea... |
4 | 550655668 | oviDNa_R | oviDNa | 36 | 1111 | 122 | 1111 | None | 1887226051 | Traced | False | Roughly traced | PDM15 | 301.0 | [19199, 15756, 13456] | [AOTU(R), CRE(-ROB,-RUB)(R), CRE(R), EPA(R), I... | [CRE(-ROB,-RUB)(R), CRE(R), INP, SCL(R), SIP(R... | {'SNP(R)': {'pre': 14, 'post': 772, 'downstrea... |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
96 | 5813068915 | NPFP1(PDM12)_L | NPFP1 | 123 | 108 | 579 | 108 | None | 4221447995 | Traced | False | Roughly traced | None | 539.5 | [28242, 20366, 6808] | [ATL(L), AVLP(R), CAN(R), EPA(R), GOR(R), IB, ... | [AVLP(R), CAN(R), EPA(R), GOR(R), IB, ICL(L), ... | {'INP': {'pre': 34, 'post': 47, 'downstream': ... |
97 | 5813077405 | DNd01_L | DNd01 | 21 | 731 | 101 | 731 | None | 738596888 | Traced | False | Roughly traced | None | 342.0 | [25173, 33207, 14176] | [SMP(L), SMP(R), SNP(L), SNP(R)] | [SMP(L), SMP(R), SNP(L), SNP(R)] | {'SNP(L)': {'pre': 13, 'post': 675, 'downstrea... |
98 | 5813078134 | DNp15_R | DNp15 | 21 | 2310 | 59 | 2310 | None | 1288564222 | Traced | False | Roughly traced | None | NaN | None | [CAN(R), GNG, IPS(R), PENP, SPS(R), VLNP(R), V... | [GNG, IPS(R), VMNP] | {'VMNP': {'pre': 7, 'post': 860, 'downstream':... |
99 | 5813095193 | DNp16_R | DNp16 | 70 | 683 | 429 | 683 | None | 292910198 | Traced | False | Roughly traced | PVM02 | 276.0 | [25941, 10730, 20000] | [CAN(R), GNG, IB, INP, IPS(R), PENP, SPS(R), V... | [CAN(R), GNG, IPS(R), PENP, SPS(R), VMNP] | {'INP': {'post': 34, 'upstream': 34}, 'IB': {'... |
100 | 5813108230 | DNp16/17_R | DNp16/17 | 5 | 177 | 16 | 177 | None | 304268206 | Traced | False | Roughly traced | PVM02 | 321.5 | [23677, 10111, 18544] | [GNG, IB, INP, IPS(R), SPS(R), VMNP] | [IPS(R), SPS(R), VMNP] | {'INP': {'post': 2, 'upstream': 2}, 'IB': {'po... |
101 rows × 18 columns
Neuprint lets you query paths from a single source to a single target. For multi-source or -target queries, your best bet is to download the entire graph and run the queries locally using networkx or igraph.
[ ]:
# Find all paths from A PN to A DNs
paths = neu.fetch_shortest_paths(upstream_bodyId=list(map(int, ALPNs['accession']))[0],
downstream_bodyId=list(map(int, DNs['accession']))[0],
min_weight=10)
paths
path | bodyId | type | weight |
---|
So it looks like there are three separate 7-hop paths to go from M_vPNml53
to DN1a
. Let’s visualize the neurons involved!
Fetching meshes & skeletons
You can fetch skeletons as SWCs directly via neuprint-python
. For visualization however it’s easiest to load neuron morphologies via navis
. For that navis
wraps neuprint-python
and adds some convenience functions (see also the tutorial):
[ ]:
# Import the wrapped neuprint-python
# -> this exposes ALL base functions plus a couple navis-specific extras
import navis
import navis.interfaces.neuprint as neu
#client = neu.Client('https://neuprint.janelia.org', dataset='hemibrain:v1.1')
# Fetch neurons in the first path
nl = neu.fetch_skeletons(paths.loc[(paths.path == 0), 'bodyId'])
nl
Fetching: 0%| | 1/186649 [00:00<47:03:02, 1.10it/s]106979579 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/106979579?format=swc
Returned Error (400):
Key "106979579_swc" not found
200511729 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/200511729?format=swc
Returned Error (400):
Key "200511729_swc" not found
199488605 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/199488605?format=swc
Returned Error (400):
Key "199488605_swc" not found
200175008 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/200175008?format=swc
Returned Error (400):
Key "200175008_swc" not found
Fetching: 0%| | 338/186649 [00:42<4:59:29, 10.37it/s]233800675 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/233800675?format=swc
Returned Error (400):
Key "233800675_swc" not found
Fetching: 0%| | 635/186649 [01:15<5:14:24, 9.86it/s]262581649 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/262581649?format=swc
Returned Error (400):
Key "262581649_swc" not found
Fetching: 0%| | 653/186649 [01:18<6:57:46, 7.42it/s]264157789 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/264157789?format=swc
Returned Error (400):
Key "264157789_swc" not found
Fetching: 0%| | 657/186649 [01:18<5:26:54, 9.48it/s]264162026 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/264162026?format=swc
Returned Error (400):
Key "264162026_swc" not found
264170361 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/264170361?format=swc
Returned Error (400):
Key "264170361_swc" not found
Fetching: 0%| | 696/186649 [01:23<5:51:11, 8.82it/s]265181023 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/265181023?format=swc
Returned Error (400):
Key "265181023_swc" not found
265193625 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/265193625?format=swc
Returned Error (400):
Key "265193625_swc" not found
Fetching: 0%| | 772/186649 [01:31<4:38:43, 11.12it/s]266493055 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/266493055?format=swc
Returned Error (400):
Key "266493055_swc" not found
Fetching: 1%| | 989/186649 [01:58<5:24:44, 9.53it/s]268272304 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/268272304?format=swc
Returned Error (400):
Key "268272304_swc" not found
Fetching: 1%| | 1062/186649 [02:06<4:56:26, 10.43it/s]269269277 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/269269277?format=swc
Returned Error (400):
Key "269269277_swc" not found
Fetching: 1%| | 1075/186649 [02:07<4:07:48, 12.48it/s]269631563 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/269631563?format=swc
Returned Error (400):
Key "269631563_swc" not found
Fetching: 1%| | 1097/186649 [02:09<5:28:30, 9.41it/s]270425747 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/270425747?format=swc
Returned Error (400):
Key "270425747_swc" not found
Fetching: 1%| | 1318/186649 [02:33<4:19:38, 11.90it/s]293625424 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/293625424?format=swc
Returned Error (400):
Key "293625424_swc" not found
293690016 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/293690016?format=swc
Returned Error (400):
Key "293690016_swc" not found
Fetching: 1%| | 1325/186649 [02:34<4:19:16, 11.91it/s]294411014 generated an exception: Error accessing GET https://neuprint.janelia.org/api/skeletons/skeleton/hemibrain:v1.1/294411014?format=swc
Returned Error (400):
Key "294411014_swc" not found
Fetching: 1%| | 1511/186649 [03:01<5:46:30, 8.90it/s]
---------------------------------------------------------------------------
KernelInterrupted: Execution interrupted by the Jupyter kernel.
[ ]:
# Let's also get some ROI meshes
al = neu.fetch_roi('AL(R)')
lh = neu.fetch_roi('LH(R)')
ca = neu.fetch_roi('CA(R)')
[ ]:
# Plot
navis.plot3d([nl, lh, al, ca], width=1100)